编写一个row套接字,实现udp数据接收
时间: 2024-02-03 19:03:37 浏览: 19
以下是使用 raw socket 实现 UDP 数据接收的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <netinet/ip.h>
#include <netinet/udp.h>
#define BUFFER_SIZE 65536
int main() {
int sockfd;
char buffer[BUFFER_SIZE];
struct sockaddr_in servaddr;
int len;
sockfd = socket(AF_INET, SOCK_RAW, IPPROTO_UDP);
if (sockfd < 0) {
perror("socket creation failed");
exit(EXIT_FAILURE);
}
memset(&servaddr, 0, sizeof(servaddr));
servaddr.sin_family = AF_INET;
servaddr.sin_addr.s_addr = INADDR_ANY;
servaddr.sin_port = htons(8888);
if (bind(sockfd, (struct sockaddr *) &servaddr, sizeof(servaddr)) < 0) {
perror("bind failed");
exit(EXIT_FAILURE);
}
printf("Waiting for UDP packets...\n");
while (1) {
len = recvfrom(sockfd, buffer, BUFFER_SIZE, 0, NULL, NULL);
if (len < 0) {
perror("recvfrom failed");
exit(EXIT_FAILURE);
}
struct iphdr *ip = (struct iphdr *) buffer;
struct udphdr *udp = (struct udphdr *) (buffer + sizeof(struct iphdr));
printf("Received UDP packet from %s:%d to %s:%d (length=%d)\n",
inet_ntoa(*(struct in_addr *) &ip->saddr),
ntohs(udp->source),
inet_ntoa(*(struct in_addr *) &ip->daddr),
ntohs(udp->dest),
len - sizeof(struct iphdr));
// 处理 UDP 数据包
// ...
// 清空缓冲区
memset(buffer, 0, BUFFER_SIZE);
}
close(sockfd);
return 0;
}
```
在这个示例中,我们使用 `socket(AF_INET, SOCK_RAW, IPPROTO_UDP)` 创建了一个 raw socket,并绑定到了本地的 8888 端口上。在 `recvfrom()` 函数中,使用 `NULL` 参数来忽略源地址和端口号。然后,我们可以使用 `struct iphdr` 和 `struct udphdr` 结构体来解析接收到的 UDP 数据包,并对数据包进行处理。注意,因为 raw socket 可以接收到所有的 IP 数据包,因此需要在代码中进行过滤,只处理 UDP 数据包。
相关推荐
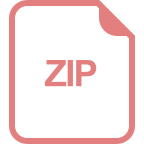
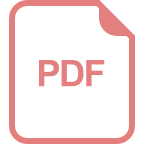














