datagridview重写实现treeview功能
时间: 2024-10-14 22:09:57 浏览: 20
DataGridView 和 TreeView 都是 Windows Forms 控件,用于显示数据集的不同视图。通常情况下,DataGridView 是用来展示表格形式的数据,而 TreeView 则更适合树状结构的数据展示。
如果你想要实现类似 DataGridView 的功能,但用 TreeView 显示数据,可以通过自定义 TreeNode 类来模拟行、列和单元格的行为。以下是一个简化的步骤:
1. **创建数据模型**:首先,你需要有一个可以存储和表示数据的类(比如 `DataGridViewRow` 对应的 `TreeNode`)。
```csharp
public class CustomTreeNode : TreeNode
{
public List<string> Columns { get; set; }
public string Value { get; set; }
// 构造函数和必要的属性设置
}
```
2. **填充数据到 CustomTreeNode**:遍历 DataGridView 的行数据,将其转换为 CustomTreeNode 并添加到 TreeView 中。
```csharp
foreach (DataGridViewRow row in dataGridView1.Rows)
{
CustomTreeNode node = new CustomTreeNode();
node.Columns = new List<string>(row.Cells.Cast<DataGridViewCell>().Select(cell => cell.Value.ToString()));
node.Text = row.Cells[0].Value.ToString(); // 根据实际需求选择标题列
treeView1.Nodes.Add(node);
}
```
3. **处理选中的 CustomTreeNode**:为了实现单元格点击的效果,你可以重写 TreeNode 的 `MouseClick` 或者 `NodeMouseClick` 方法,根据节点位置获取对应的单元格值。
```csharp
node.NodeMouseClick += (sender, e) =>
{
if (e.Button == MouseButtons.Left)
{
// 获取当前单元格索引和值
int columnIndex = Convert.ToInt32(e.Location.X / dataGridView1.Width) + 1;
string cellValue = node.SubItems[columnIndex - 1].Text;
// 执行相应的操作,如更新界面或通知事件
}
};
```
4. **滚动和分页支持**:虽然默认的 TreeView 没有直接的分页或滚动功能,但可以通过手动管理 TreeNode 的层级和宽度来模拟这种效果。
阅读全文
相关推荐
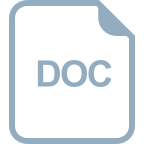
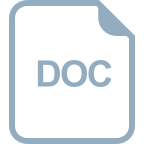
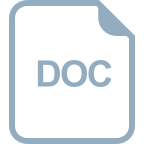

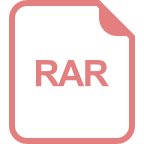
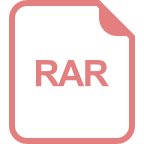
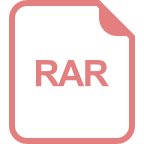
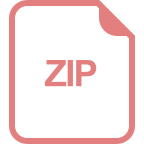
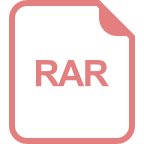
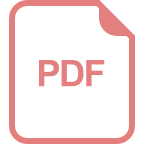
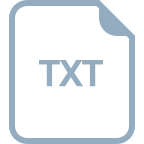
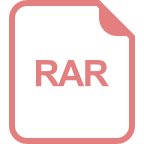
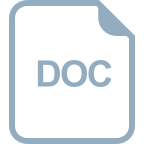
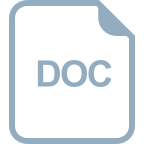

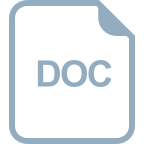
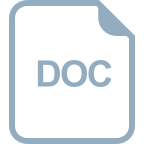