random sample函数
时间: 2023-11-19 09:53:49 浏览: 86
random sample函数是Python中的一个随机函数,其函数原型为random.sample(sequence, k),用于从指定序列中随机获取指定长度的片断。其中,sequence表示指定的序列,k表示需要获取的片断长度。需要注意的是,如果k大于sequence元素个数的话会报错。此函数不会修改原有序列。
相关问题
在 C++ 中,对实时点云进行随机采样 pcl::RandomSample 函数
在 C++ 中,对实时点云进行随机采样可以使用 `pcl::RandomSample` 函数。如果需要对实时点云进行随机采样,可以使用 `pcl::ExtractIndices` 过滤器结合 `pcl::RandomSample` 函数实现。具体的流程如下:
1. 定义 `pcl::PointCloud` 用于存储实时点云数据,定义 `pcl::PointCloud` 用于存储随机采样后的点云数据。
```cpp
pcl::PointCloud<pcl::PointXYZ>::Ptr input_cloud(new pcl::PointCloud<pcl::PointXYZ>);
pcl::PointCloud<pcl::PointXYZ>::Ptr output_cloud(new pcl::PointCloud<pcl::PointXYZ>);
```
2. 在回调函数中读取实时点云数据,并将数据存储在 `input_cloud` 中。
```cpp
void cloud_callback(const pcl::PointCloud<pcl::PointXYZ>::ConstPtr& input)
{
*input_cloud = *input;
}
```
3. 将 `input_cloud` 中的点随机打乱。
```cpp
std::random_shuffle(input_cloud->points.begin(), input_cloud->points.end());
```
4. 使用 `pcl::RandomSample` 函数从打乱后的点云中随机选择指定数量的点,并将结果存储在 `output_cloud` 中。
```cpp
pcl::RandomSample<pcl::PointXYZ> random_sample;
random_sample.setInputCloud(input_cloud);
random_sample.setSample(sample_size);
random_sample.filter(*output_cloud);
```
5. 将随机采样后的点云数据发布出去。
```cpp
ros::Publisher pub = nh.advertise<pcl::PointCloud<pcl::PointXYZ>>("output", 1);
pub.publish(output_cloud);
```
完整的代码示例如下:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
#include <ros/ros.h>
#include <pcl/point_cloud.h>
#include <pcl/point_types.h>
#include <pcl/filters/extract_indices.h>
#include <pcl/filters/random_sample.h>
#include <pcl_ros/point_cloud.h>
#include <sensor_msgs/PointCloud2.h>
pcl::PointCloud<pcl::PointXYZ>::Ptr input_cloud(new pcl::PointCloud<pcl::PointXYZ>);
pcl::PointCloud<pcl::PointXYZ>::Ptr output_cloud(new pcl::PointCloud<pcl::PointXYZ>);
void cloud_callback(const pcl::PointCloud<pcl::PointXYZ>::ConstPtr& input)
{
*input_cloud = *input;
// 随机打乱点云中的点
std::random_shuffle(input_cloud->points.begin(), input_cloud->points.end());
// 随机采样点云
int sample_size = 1000;
pcl::RandomSample<pcl::PointXYZ> random_sample;
random_sample.setInputCloud(input_cloud);
random_sample.setSample(sample_size);
random_sample.filter(*output_cloud);
// 发布随机采样后的点云数据
ros::NodeHandle nh;
ros::Publisher pub = nh.advertise<pcl::PointCloud<pcl::PointXYZ>>("output", 1);
pub.publish(output_cloud);
}
int main(int argc, char** argv)
{
ros::init(argc, argv, "random_sample");
ros::NodeHandle nh;
// 订阅原始点云数据
ros::Subscriber sub = nh.subscribe<pcl::PointCloud<pcl::PointXYZ>>("input", 1, cloud_callback);
ros::spin();
return 0;
}
```
需要注意的是,以上代码仅仅是示例代码,实际应用中需要根据具体的需求进行修改。
random.sample函数
random.sample函数是Python标准库中的一个函数,它用于从指定的序列中随机选择指定数量的元素并返回一个新的列表。该函数的语法如下:
random.sample(sequence, k)
其中,sequence是要进行随机选择的序列,可以是列表、元组、字符串等可迭代对象;k是要选择的元素数量。
示例用法:
```python
import random
my_list = [1, 2, 3, 4, 5]
sampled_list = random.sample(my_list, 3)
print(sampled_list)
```
以上代码会从`my_list`中随机选择3个元素,并将结果存储在`sampled_list`中输出。注意,被选中的元素不会重复。
希望能够帮到你!如果还有其他问题,请继续提问。
阅读全文
相关推荐
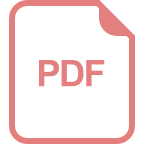













