用VUE3帮我写一个轮播图
时间: 2024-06-13 20:05:24 浏览: 19
好的,以下是一个使用Vue3实现轮播图的示例代码:
```
<template>
<div class="carousel">
<div class="carousel-inner" :style="{transform: 'translateX(' + translateX + 'px)'}">
<div class="carousel-item" v-for="(item, index) in items" :key="index">
<img :src="item.src" alt="">
</div>
</div>
<div class="carousel-dots">
<span class="dot" v-for="(item, index) in items" :key="index" :class="{active: currentIndex === index}" @click="goTo(index)"></span>
</div>
</div>
</template>
<script>
import { ref, watch } from 'vue';
export default {
name: 'Carousel',
props: {
items: {
type: Array,
required: true
},
interval: {
type: Number,
default: 3000
}
},
setup(props) {
const currentIndex = ref(0);
const translateX = ref(0);
const timer = ref(null);
const goTo = (index) => {
currentIndex.value = index;
translateX.value = -index * 100;
resetTimer();
};
const next = () => {
currentIndex.value = (currentIndex.value + 1) % props.items.length;
translateX.value = -currentIndex.value * 100;
};
const resetTimer = () => {
if (timer.value) {
clearInterval(timer.value);
}
timer.value = setInterval(next, props.interval);
};
watch(currentIndex, resetTimer);
resetTimer();
return {
currentIndex,
translateX,
goTo
};
}
};
</script>
<style>
.carousel {
position: relative;
width: 100%;
height: 300px;
overflow: hidden;
}
.carousel-inner {
display: flex;
transition: transform 0.5s ease;
}
.carousel-item {
flex: 0 0 100%;
}
.carousel-item img {
width: 100%;
height: 100%;
object-fit: cover;
}
.carousel-dots {
position: absolute;
bottom: 20px;
left: 50%;
transform: translateX(-50%);
display: flex;
}
.dot {
width: 10px;
height: 10px;
border-radius: 50%;
background-color: #ccc;
margin-right: 10px;
cursor: pointer;
}
.dot.active {
background-color: #333;
}
</style>
```
这个轮播图组件接受一个items数组作为轮播图的数据源,interval属性表示轮播间隔时间,默认为3秒。你可以在父组件中使用这个组件,并传入相应的数据源和属性,例如:
```
<template>
<div>
<carousel :items="items" :interval="3000"></carousel>
</div>
</template>
<script>
import Carousel from './Carousel.vue';
export default {
name: 'App',
components: {
Carousel
},
data() {
return {
items: [
{ src: 'https://picsum.photos/id/1018/800/600' },
{ src: 'https://picsum.photos/id/1015/800/600' },
{ src: 'https://picsum.photos/id/1019/800/600' },
{ src: 'https://picsum.photos/id/1020/800/600' }
]
};
}
};
</script>
```
这个示例中,我们传入了一个包含4张图片的数组作为轮播图的数据源。你可以根据自己的需求修改数据源和样式。
相关推荐
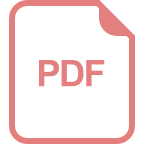
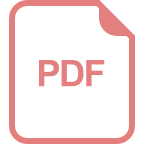
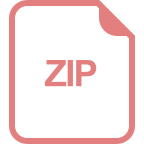
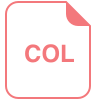
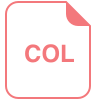
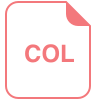
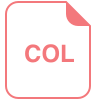
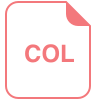









