vue3.2对象赋值
时间: 2023-09-22 21:15:06 浏览: 232
在Vue 3.2中,可以使用以下语法来进行对象赋值:
1. 在Vue组件中,可以使用`v-model`指令将一个数据对象绑定到一个表单输入元素上,例如:
```html
<template>
<input v-model="myObject.name" type="text">
</template>
```
这里的`myObject`是一个在Vue实例的`data`选项中定义的对象。
2. 可以使用`this.$set`方法来给对象添加新的属性,例如:
```javascript
this.$set(this.myObject, 'age', 25);
```
这里的`myObject`是在Vue实例的`data`选项中定义的对象。
3. 可以使用`Object.assign`方法来合并对象,例如:
```javascript
this.myObject = Object.assign({}, this.myObject, { age: 25 });
```
这里的`myObject`是在Vue实例的`data`选项中定义的对象。
请注意,以上方法都是在Vue组件中使用的。如果在VueX中进行对象赋值,可以使用类似的语法。
以上是关于Vue 3.2中对象赋值的一些示例方法,具体的使用方式取决于你的具体需求和代码结构。
相关问题
vue3.2 父页面如何获取子组件的实例
在 Vue.js 3.2 或更高版本中,您可以使用 `ref` 属性在父组件中获取子组件的实例。
首先,在子组件中添加 `ref` 属性,并将其命名为一个合适的变量名,例如 `myComponent`:
```html
<template>
<div ref="myComponent">
<!-- 子组件的模板代码 -->
</div>
</template>
```
然后,在父组件中使用 `ref` 属性获取子组件的实例。在父组件的 `mounted` 生命周期钩子函数中,通过 `$refs` 对象访问子组件的实例:
```html
<template>
<div>
<!-- 父组件的模板代码 -->
<button @click="getComponentInstance">获取子组件实例</button>
</div>
</template>
<script>
export default {
mounted() {
this.$refs.myComponent.doSomething(); // 在父组件中调用子组件的方法
},
methods: {
getComponentInstance() {
const childComponent = this.$refs.myComponent;
console.log(childComponent); // 打印子组件实例
},
},
}
</script>
```
上面的例子中,我们在父组件中使用 `$refs.myComponent` 访问子组件的实例。在 `getComponentInstance` 方法中,我们将子组件的实例赋值给一个变量 `childComponent`,并打印出来。您可以在这个方法中做任何您想要的事情,比如调用子组件的方法、监听子组件的事件,等等。
vue3.2中使用setup语法糖如何用reactive
在Vue 3.2中使用`setup`语法糖,可以通过`reactive`函数将一个普通对象转换为响应式对象。`reactive`函数返回的是一个代理对象,这个代理对象会将访问和修改映射到原始对象上。
下面是一个使用`reactive`函数的示例:
```html
<template>
<div>{{ state.count }}</div>
</template>
<script>
import { reactive } from 'vue';
export default {
setup() {
const state = reactive({
count: 0,
});
return { state };
},
};
</script>
```
在上面的示例中,我们定义了一个名为`state`的响应式对象,它包含一个`count`属性。在`setup`函数中,我们使用`reactive`函数将一个普通对象转换为响应式对象,并将其赋值给`state`变量。最后,我们将`state`变量作为返回值,使得组件内可以使用它。
需要注意的是,在`setup`函数内部,不能直接访问组件实例(`this`),因为`setup`函数在组件实例创建之前就已经执行了。如果需要访问组件实例,可以通过`getCurrentInstance`函数获取当前的组件实例。
阅读全文
相关推荐
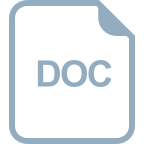
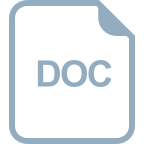
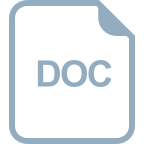
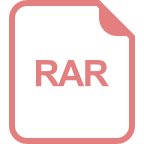
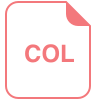
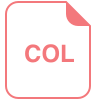
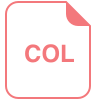
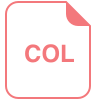
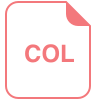
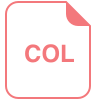
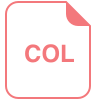
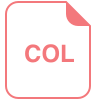
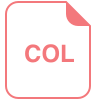
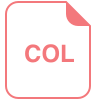
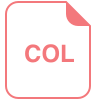
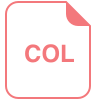
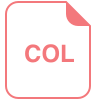