它需要生成C:\Users\陈芃宇\Downloads\汉字字库.txt这个文本文档里面的字符的图片
时间: 2023-08-23 21:05:24 浏览: 43
好的,您想要使用文本文档`C:\Users\陈芃宇\Downloads\汉字字库.txt`中的字符生成图片,以下是一个示例代码:
```python
from PIL import Image, ImageDraw, ImageFont
def text_to_image(text, font_path, font_size, image_size, image_color, text_color):
# 创建空白图片
image = Image.new('RGB', image_size, color=image_color)
draw = ImageDraw.Draw(image)
# 加载字体
font = ImageFont.truetype(font_path, font_size)
# 计算文字绘制位置
text_width, text_height = draw.textsize(text, font=font)
x = (image_size[0] - text_width) // 2
y = (image_size[1] - text_height) // 2
# 绘制文字
draw.text((x, y), text, font=font, fill=text_color)
return image
# 批量转换文字为图片
def batch_convert_text_to_image(texts, font_path, font_size, image_size, image_color, text_color):
for i, text in enumerate(texts):
image = text_to_image(text, font_path, font_size, image_size, image_color, text_color)
image.save(f'output_{i}.png')
# 从文本文件中读取字符
def read_characters_from_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
characters = file.read().strip()
return characters
# 示例用法
file_path = r'C:\Users\陈芃宇\Downloads\汉字字库.txt'
font_path = 'Arial.ttf' # 字体文件路径
font_size = 24 # 字体大小
image_size = (200,100) # 图片大小
image_color = (255, 255, 255) # 图片背景颜色(RGB)
text_color = (0, 0, 0) # 文字颜色(RGB)
# 读取文本文件中的字符
characters = read_characters_from_file(file_path)
# 批量转换字符为图片
batch_convert_text_to_image(characters, font_path, font_size, image_size, image_color, text_color)
```
请将`file_path`变量设置为您的文本文档的路径(例如:`C:\Users\陈芃宇\Downloads\汉字字库.txt`)。代码将读取文档中的字符,并根据提供的字体、大小、图片大小等信息,生成相应的图片,并保存在当前目录下,文件名为`output_0.png`、`output_1.png`、`output_2.png`等。
请确保安装了Pillow库,可以使用`pip install pillow`命令进行安装。同时,您需要提供所需的字体文件(`font_path`)的路径。
相关推荐
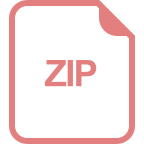
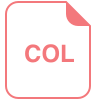
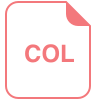
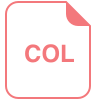
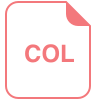










