HttpServletResponse重定向到html 404
时间: 2023-08-18 11:13:37 浏览: 220
您可以使用 `HttpServletResponse` 的 `sendRedirect` 方法将请求重定向到一个 HTML 页面,并返回 404 状态码。以下是一个示例代码:
```java
response.sendRedirect("/404.html");
response.setStatus(HttpServletResponse.SC_NOT_FOUND);
```
在这个示例中,`/404.html` 是您自定义的 HTML 页面路径。当调用 `sendRedirect` 方法时,客户端将被重定向到该页面,并且 `setStatus` 方法设置了响应状态码为 404。
请注意,您需要确保在您的服务器上存在这个 HTML 页面,以便能够正确地进行重定向。
相关问题
HttpServletResponse重定向到html
您可以使用`HttpServletResponse`的`sendRedirect()`方法将请求重定向到HTML页面。示例如下:
```java
String redirectUrl = "http://example.com/mypage.html";
response.sendRedirect(redirectUrl);
```
在上述代码中,您需要将`redirectUrl`替换为您要重定向的HTML页面的URL。当客户端收到重定向响应后,将自动跳转到指定的HTML页面。
请注意,如果要重定向到同一应用程序中的相对路径的HTML页面,则可以使用相对路径而不是完整的URL。例如,如果您要重定向到位于应用程序根目录下的`mypage.html`,则可以使用以下代码:
```java
String redirectUrl = "/mypage.html";
response.sendRedirect(redirectUrl);
```
这样,重定向将在相对路径的基础上发生,而不是跳转到完整的URL。
java web请按照以下要求编写程序。 1.编写用户登录的界面login.html和登录成功的界面welcome.html。 2.编写处理用户登录请求的Servlet类Login。 3.Login类中判断表单中如果输入的用户名为“itcast”,密码为“itcast”,将请求重定向到welcome.html页面,否则重定向到login.html页面。
好的,以下是代码实现:
1. login.html
```html
<!DOCTYPE html>
<html>
<head>
<title>Login</title>
</head>
<body>
<form action="login" method="post">
<label>Username:</label>
<input type="text" name="username"><br>
<label>Password:</label>
<input type="password" name="password"><br>
<input type="submit" value="Login">
</form>
</body>
</html>
```
2. welcome.html
```html
<!DOCTYPE html>
<html>
<head>
<title>Welcome</title>
</head>
<body>
<h1>Welcome!</h1>
</body>
</html>
```
3. Login Servlet
```java
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/login")
public class Login extends HttpServlet {
private static final long serialVersionUID = 1L;
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
if (username.equals("itcast") && password.equals("itcast")) {
response.sendRedirect("welcome.html");
} else {
response.sendRedirect("login.html");
}
}
}
```
在这个Servlet中,我们首先获取表单中的用户名和密码,然后判断是否为“itcast”,如果是,将请求重定向到welcome.html页面,否则重定向到login.html页面。注意,我们在@WebServlet注解中指定了Servlet的访问路径为“/login”。
阅读全文
相关推荐
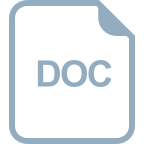
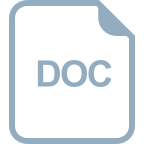
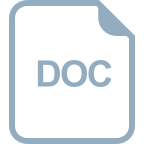
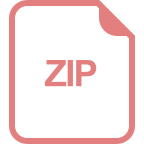
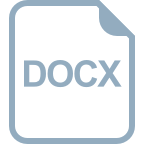











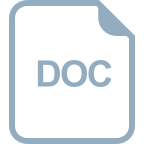