用c语言编程模拟进程管理,要有创建新的进程,查看运行进程,换出某个进程,杀死运行进程以及进程之间通信功能写出代码
时间: 2024-03-01 14:51:56 浏览: 87
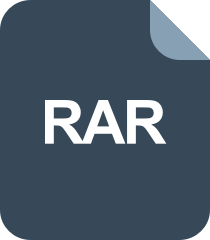
模拟进程管理c语言至少要有:创建新的进程;查看运行进程;换出某个进程;杀死运行进程以及进程之间通信等功能。

下面是一个简单的C语言程序,用于模拟进程管理,包括创建新的进程,查看运行进程,换出某个进程,杀死运行进程以及进程之间通信功能:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
#define BUFFER_SIZE 25
#define READ_END 0
#define WRITE_END 1
int main(int argc, char *argv[]) {
//创建管道
char write_msg[BUFFER_SIZE] = "Hello, world!";
char read_msg[BUFFER_SIZE];
int fd[2];
pid_t pid;
if (pipe(fd) == -1) {
fprintf(stderr, "Pipe failed");
return 1;
}
//创建子进程
pid = fork();
if (pid < 0) {
fprintf(stderr, "Fork failed");
return 1;
}
if (pid == 0) {
//子进程
close(fd[READ_END]);
//写入数据到管道
write(fd[WRITE_END], write_msg, strlen(write_msg)+1);
close(fd[WRITE_END]);
}
else {
//父进程
close(fd[WRITE_END]);
//读取数据从管道
read(fd[READ_END], read_msg, BUFFER_SIZE);
printf("Received message: %s\n", read_msg);
close(fd[READ_END]);
}
return 0;
}
```
以上代码实现了基本的进程管道通信。您可以根据具体需求进行修改和扩展。
阅读全文
相关推荐


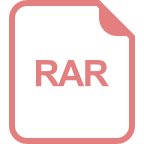
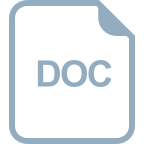
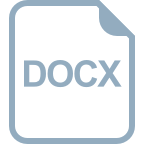
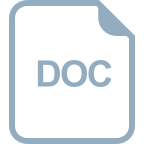
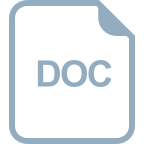
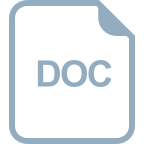
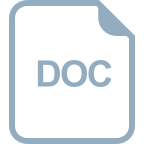
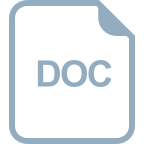
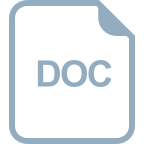
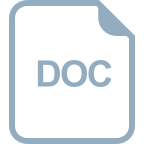
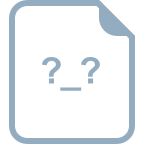
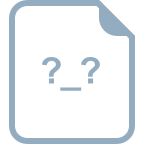