springboot+easypoi导出时,遍历需要导出的数据,与用户所选择需要导出的字段进行匹配,若不匹配则隐藏或删除该列,需要注意的是一对多这种情况,操作中可能会出现隐藏失败,请给我详细代码和步骤
时间: 2024-05-15 12:17:31 浏览: 106
好的,针对您的问题,可以先使用 EasyPoi 提供的工具类 ExcelExportUtil.exportExcelBig 方法来导出 Excel 文件。该方法有如下参数:
```
/**
* ExcelExportUtil 导出 Excel 的大数据量方法
*
* @param params 参数对象
* isCreateHeader: 是否创建表头
* title: 表头标题
* sheetName: 工作表名称
* pojoClass: 实体类类型
* dataSet: 导出数据集合
* file: 保存 Excel 的文件对象
*/
public static <T> void exportExcelBig(Map<String, Object> params, Class<T> pojoClass, Collection<T> dataSet, File file) throws Exception {
...
}
```
其中,最后一个参数 "file" 代表导出的 Excel 文件对象。
在导出之前,需要先根据用户选择的需要导出的字段进行匹配,得到最终要导出的字段信息。对于一对多的情况,可以使用 EasyPoi 的 @ExcelCollection 注解来标记一个集合属性,并在需要导出的实体类中添加一个对应的集合属性,例如:
```
public class OrderExportVo {
// 订单编号
@Excel(name = "订单编号", width = 20)
private String orderNo;
// 订单时间
@Excel(name = "订单时间", width = 20)
private Date orderTime;
// 商品列表
@ExcelCollection(name = "商品列表")
private List<ProductExportVo> productList;
}
public class ProductExportVo {
// 商品编号
@Excel(name = "商品编号", orderNum = "1", width = 20)
private String productNo;
// 商品名称
@Excel(name = "商品名称", orderNum = "2", width = 20)
private String productName;
// 商品价格
@Excel(name = "商品价格", orderNum = "3", width = 20)
private Double price;
}
```
其中,OrderExportVo 对应订单信息,ProductExportVo 对应商品信息。OrderExportVo 中的 productList 属性标记了 @ExcelCollection 注解,表明该属性是一个商品列表,并且在导出时需要递归导出商品列表中的每一个商品信息。
最终导出时,可以使用以下代码:
```
// 1. 根据用户选择的需要导出的字段,得到最终要导出的字段信息,并存入 List<Field> fields 即可
// 2. 设置表头和数据属性
Map<String, Object> params = new HashMap<>();
params.put(NormalExcelConstants.CLASS, OrderExportVo.class);
params.put(NormalExcelConstants.PARAMS, new ExportParams(null, "商品订单表"));
// 3. 将需要导出的数据集合 dataSet 转成要导出的实体类对象集合
List<OrderExportVo> orderExportVos = new ArrayList<>();
for (Order order : orders) {
OrderExportVo orderExportVo = new OrderExportVo();
orderExportVo.setOrderNo(order.getOrderNo());
orderExportVo.setOrderTime(order.getOrderTime());
List<ProductExportVo> productExportVos = new ArrayList<>();
for (Product product : order.getProducts()) {
ProductExportVo productExportVo = new ProductExportVo();
productExportVo.setProductNo(product.getProductNo());
productExportVo.setProductName(product.getProductName());
productExportVo.setPrice(product.getPrice());
productExportVos.add(productExportVo);
}
orderExportVo.setProductList(productExportVos);
orderExportVos.add(orderExportVo);
}
// 4. 遍历需要导出的实体类对象集合,将不需要导出的属性值设置为 null
for (OrderExportVo orderExportVo : orderExportVos) {
for (Field field : fields) {
if (!field.getName().equals("productList")) { // 普通属性
try {
Object value = BeanUtils.getProperty(orderExportVo, field.getName());
if (value != null && StringUtils.isBlank(value.toString())) {
BeanUtils.setProperty(orderExportVo, field.getName(), null);
}
} catch (Exception e) {
log.error(e.getMessage(), e);
}
} else { // 集合属性
List<ProductExportVo> productList = orderExportVo.getProductList();
if (CollectionUtils.isNotEmpty(productList)) {
for (ProductExportVo productExportVo : productList) {
for (Field f : productExportVo.getClass().getDeclaredFields()) {
if (!fields.contains(f)) {
try {
Object value = BeanUtils.getProperty(productExportVo, f.getName());
if (value != null && StringUtils.isBlank(value.toString())) {
BeanUtils.setProperty(productExportVo, f.getName(), null);
}
} catch (Exception e) {
log.error(e.getMessage(), e);
}
}
}
}
}
}
}
}
// 5. 导出 Excel,传入需要导出的实体类对象集合
ExcelExportUtil.exportExcel(params, fields, orderExportVos, file));
```
需要注意的是,在遍历需要导出的实体类对象集合时,需要将不需要导出的属性值设置为 null。另外,导出 Excel 时,需要传入 List<Field> 类型的字段列表,用于在导出时根据需要导出的字段顺序来生成表头。
阅读全文
相关推荐
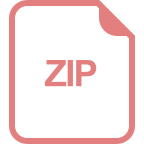
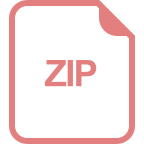
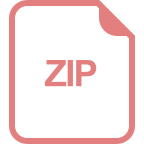














