在vue中input框得到焦点选中文字
时间: 2024-02-27 10:56:42 浏览: 80
您好!在 Vue 中,可以通过 `$refs` 来获取输入框元素,从而实现在输入框获取焦点时自动选中文本的效果。
具体实现步骤如下:
1. 在 input 元素上添加 `ref` 属性,以便在 Vue 实例中获取该元素。
```html
<template>
<div>
<input type="text" ref="input" v-model="text" @focus="selectText">
</div>
</template>
```
2. 在 Vue 实例中定义 `selectText` 方法,该方法在 input 元素获得焦点时自动选中文本。
```javascript
export default {
data() {
return {
text: 'Hello World',
};
},
methods: {
selectText() {
this.$refs.input.select();
},
},
};
```
通过调用 `this.$refs.input.select()` 方法,就可以实现在 input 元素获取焦点时自动选中文本的效果。
希望对您有所帮助!
相关问题
在vue中获取input 焦点
首先,在Vue中,获取input焦点的方法可以有多种,以下是其中的一些方法:
1. 使用ref属性获取input元素,并在mounted生命周期钩子中将焦点设置在该元素上:
```html
<template>
<div>
<input type="text" ref="myInput">
</div>
</template>
<script>
export default {
mounted() {
this.$refs.myInput.focus();
}
}
</script>
```
2. 通过v-if指令来判断是否需要显示该input元素,并使用Vue的nextTick方法在DOM更新后将焦点设置在该元素上:
```html
<template>
<div>
<input type="text" v-if="showInput" @focus="onFocus">
</div>
</template>
<script>
export default {
data() {
return {
showInput: true
}
},
methods: {
onFocus() {
this.$nextTick(() => {
this.$refs.myInput.focus();
});
}
}
}
</script>
```
3. 使用自定义指令来设置input元素的焦点:
```html
<template>
<div>
<input type="text" v-focus>
</div>
</template>
<script>
export default {
directives: {
focus: {
inserted(el) {
el.focus();
}
}
}
}
</script>
```
以上是一些常用的获取input焦点的方法,具体使用哪种方法,可以根据自己的实际需求来选择。
ant-degin-vue的input框内输入内容回车或离开input框,输入的内容变成tag标签必须在input框内并且最多只能输入十个标签,超过十个则不能输入任何东西
你可以使用Ant Design Vue提供的`Input`和`Tag`组件来实现这个功能。下面是一个简单的例子:
```vue
<template>
<div>
<a-tag
v-for="(tag, index) in tags"
:key="index"
closable
@close="handleClose(tag)"
>
{{ tag }}
</a-tag>
<a-input
ref="input"
v-model="inputValue"
@keydown.enter="handleEnter"
@blur="handleBlur"
/>
</div>
</template>
<script>
export default {
data() {
return {
tags: [],
inputValue: ""
};
},
methods: {
handleClose(tag) {
this.tags = this.tags.filter(t => t !== tag);
},
handleEnter() {
const tag = this.inputValue.trim();
if (tag && this.tags.length < 10) {
this.tags.push(tag);
this.inputValue = "";
}
},
handleBlur() {
this.handleEnter();
if (this.tags.length === 10) {
this.$refs.input.$el.blur();
}
}
}
};
</script>
```
在上面的例子中,我们使用了`v-for`指令来渲染已选择的标签,使用`closable`属性来显示关闭按钮。我们使用`v-model`指令来双向绑定输入框的值,使用`@keydown.enter`事件来处理回车事件,使用`@blur`事件来处理失去焦点事件。
在`handleEnter`方法中,我们首先获取输入框的值,并去除首尾的空格。然后判断输入框的值是否为空,以及已选择的标签数量是否超过了10个。如果满足条件,就将输入框的值添加到已选择的标签中,并清空输入框的值。
在`handleBlur`方法中,我们首先调用`handleEnter`方法来处理输入框的值。然后判断已选择的标签数量是否达到了10个,如果是,就让输入框失去焦点。
这样,就实现了在输入框内输入内容回车或离开input框,输入的内容变成tag标签,并且最多只能输入十个标签,超过十个则不能输入任何东西的功能。
阅读全文
相关推荐


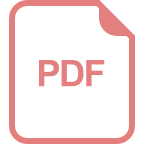
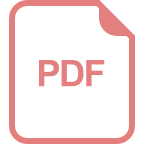
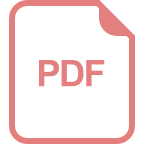



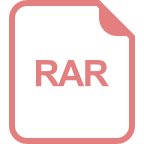
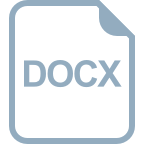
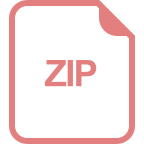
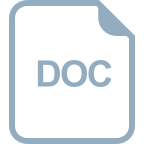
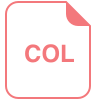