python词频统计西游记
时间: 2024-06-03 12:06:32 浏览: 340
Python中可以使用多种方法进行词频统计,常用的是使用Python内置库collections的Counter类。下面是一个示例代码,可以统计《西游记》中出现频率最高的前20个词语及其出现次数:
```
import jieba
from collections import Counter
# 读取文本文件
with open('journey_to_the_west.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 使用jieba分词
words = jieba.cut(text)
# 统计词频
counter = Counter(words)
top_words = counter.most_common(20)
# 输出结果
for word, count in top_words:
print(f'{word}: {count}')
```
需要注意的是,在运行代码前需要安装jieba库,可以使用以下命令进行安装:
```
pip install jieba
```
另外,需要将《西游记》的文本文件(journey_to_the_west.txt)放在与代码同一目录下。
相关问题
python西游记词频统计
以下是Python实现《西游记》词频统计的代码和步骤:
1. 读取《西游记》文本文件
```python
with open('xyj.txt', 'r', encoding='utf-8') as f:
text = f.read()
```
2. 使用正则表达式去除文本中的非汉字字符
```python
import re
text = re.sub('[^\u4e00-\u9fa5]', '', text)
```
3. 统计每个汉字出现的次数
```python
from collections import Counter
counter = Counter(text)
```
4. 统计不同汉字的个数
```python
num_unique_chars = len(counter)
```
5. 找出出现次数最多的前10个汉字
```python
most_common = counter.most_common(10)
```
6. 将结果保存到json文件中
```python
import json
result = {
'num_unique_chars': num_unique_chars,
'most_common': most_common,
'counter': dict(counter)
}
with open('result.json', 'w', encoding='utf-8') as f:
json.dump(result, f, ensure_ascii=False, indent=4)
```
python西游记词频统计代码
Python西游记词频统计代码可以用Python的内置库collections来实现。以下是一个简单的示例:
```
import collections
# 读取文件内容
with open('journey_to_the_west.txt', 'r', encoding='utf-8') as f:
content = f.read()
# 统计词频
words = content.split()
word_counts = collections.Counter(words)
# 输出前10个高频词
for word, count in word_counts.most_common(10):
print(word, count)
```
在这个代码中,我们使用了Python内置的Counter类来统计词频。首先,我们打开journey_to_the_west.txt文件,并将其内容读取到content变量中。然后,我们将content按照空格划分为单词,并使用Counter统计单词出现的次数。最后,我们使用most_common方法找出出现次数最多的前10个单词,并将它们输出到屏幕上。
如果你想要深入学习Python的文本处理功能,我建议你学习Python的nltk和spaCy等第三方库。这些库提供了更强大的文本处理功能,可以帮助你更好地完成类似的任务。
阅读全文
相关推荐
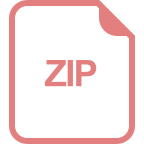











