多层感知机的正向传播计算
时间: 2024-08-12 16:07:01 浏览: 68
多层感知机(Multilayer Perceptron, MLP)是一种前馈神经网络结构,它包含输入层、隐藏层和输出层,每个神经元与其他层的神经元相连,通过一系列非线性变换实现对复杂数据的学习。在正向传播过程中,MLP用于预测或分类任务,数据从输入层开始,经过各层直至输出层。
正向传播计算步骤如下:
1. **输入层**:原始数据作为输入传递到网络。
2. **隐藏层**:
- **激活函数应用**:每个神经元接收到输入信号后,会应用激活函数(如sigmoid、ReLU等),这个函数引入非线性,使模型能够学习复杂的模式。
- **权重和偏置**:每个神经元会乘以其连接权重加上偏置,形成加权和。
- **加权和与激活函数结合**:加权和经过激活函数处理,产生新的输出。
3. **循环隐藏层**:如果有多个隐藏层,这个过程会在每层之间重复,直至最后一个隐藏层。
4. **输出层**:最后一个隐藏层的输出进入输出层,同样通过激活函数(对于分类问题通常用softmax,对于回归用线性),然后计算预测值。
5. **误差计算**:如果这是一个训练过程,将输出层的预测结果与实际标签进行比较,计算损失函数。
6. **反向传播**:正向传播结束后,才开始反向传播更新权重,以最小化损失。这一步不在正向传播的描述中,但它与正向传播是相辅相成的。
相关问题
pytorch手写数字以PPT中具有单隐层的多层感知机及相关推导为基础,设计一个具有三个输入层节点、一个隐层(内含三个节点)、两个输出层节点的多层感知机,初始化数据参照PPT中完成,手动完成模型数据正向传播计算和权重参数反向更新计算识别
PyTorch是一个强大的深度学习库,用于构建和训练神经网络,包括多层感知机(MLPs)。在这个例子中,我们将创建一个具有三层的简单MLP,用于手写数字识别。首先,我们需要导入必要的模块:
```python
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision.datasets import MNIST
from torch.utils.data import DataLoader
```
1. **定义网络结构**:
```python
class MultiLayerPerceptron(nn.Module):
def __init__(self):
super(MultiLayerPerceptron, self).__init__()
# 输入层(3个节点),隐藏层(3个节点),输出层(2个节点)
self.fc1 = nn.Linear(784, 3) # 输入大小为28*28像素(灰度图像),每个像素作为输入节点
self.fc2 = nn.Linear(3, 3)
self.fc3 = nn.Linear(3, 2) # 输出层对应0-9共10个类别,我们选择最接近的一个
def forward(self, x):
# 隐藏层前的激活函数,这里可以用ReLU
x = nn.functional.relu(self.fc1(x))
x = nn.functional.relu(self.fc2(x))
# 输出层无激活函数,因为是分类任务,可以使用softmax
out = self.fc3(x)
return out
```
2. **加载和预处理MNIST数据集**:
```python
model = MultiLayerPerceptron()
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
model.to(device)
# 加载MNIST数据集
train_dataset = MNIST(root='./data', train=True, download=True, transform=...)
test_dataset = MNIST(root='./data', train=False, download=True, transform=...)
train_loader = DataLoader(train_dataset, batch_size=64, shuffle=True)
test_loader = DataLoader(test_dataset, batch_size=64, shuffle=False)
```
3. **定义损失函数和优化器**:
```python
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(model.parameters(), lr=0.01) # 使用随机梯度下降优化器
# 训练过程
for epoch in range(num_epochs):
for inputs, labels in train_loader:
inputs, labels = inputs.to(device), labels.to(device)
# 前向传播
outputs = model(inputs)
loss = criterion(outputs, labels)
# 反向传播并优化
optimizer.zero_grad()
loss.backward()
optimizer.step()
```
注意:以上代码简化了MNIST数据预处理的部分,实际操作需要详细处理数据归一化等步骤,并可能使用其他优化算法如Adam。此外,在训练过程中,你可以定期在验证集上评估模型性能。
**相关问题--:**
1. PyTorch的`nn.Module`是什么?
2. 为什么要对网络层应用激活函数?
3. 在训练过程中如何设置学习率调整策略?
用theano库实现卷积神经网络的反向传播
首先,我们需要定义一个卷积层的类,其中包含正向传播和反向传播的函数。
```python
import numpy as np
import theano
import theano.tensor as T
class ConvLayer(object):
def __init__(self, rng, input_shape, filter_shape):
self.input_shape = input_shape
self.filter_shape = filter_shape
fan_in = np.prod(filter_shape[1:])
fan_out = (filter_shape[0] * np.prod(filter_shape[2:]))
W_bound = np.sqrt(6. / (fan_in + fan_out))
self.W = theano.shared(
np.asarray(
rng.uniform(low=-W_bound, high=W_bound, size=filter_shape),
dtype=theano.config.floatX
),
borrow=True
)
self.b = theano.shared(
np.zeros((filter_shape[0],), dtype=theano.config.floatX),
borrow=True
)
self.params = [self.W, self.b]
def convolve(self, input):
conv_out = T.nnet.conv2d(
input=input,
filters=self.W,
filter_shape=self.filter_shape,
input_shape=self.input_shape
)
return T.nnet.sigmoid(conv_out + self.b.dimshuffle('x', 0, 'x', 'x'))
def get_cost_updates(self, cost, learning_rate):
grads = T.grad(cost, self.params)
updates = [(param, param - learning_rate * grad) for param, grad in zip(self.params, grads)]
return updates
```
接下来,我们定义一个多层感知机的类,包含训练函数和预测函数。
```python
class MLP(object):
def __init__(self, rng, input_shape, filter_shapes, hidden_sizes, output_size):
self.x = T.tensor4('x')
self.y = T.matrix('y')
self.layers = []
self.params = []
layer_input = self.x
input_shape = input_shape
for i in range(len(filter_shapes)):
filter_shape = filter_shapes[i]
layer = ConvLayer(rng=rng, input_shape=input_shape, filter_shape=filter_shape)
self.layers.append(layer)
self.params += layer.params
layer_output = layer.convolve(layer_input)
layer_input = layer_output
input_shape = (input_shape[0], filter_shape[0], input_shape[2] - filter_shape[2] + 1, input_shape[3] - filter_shape[3] + 1)
flatten_layer_output = layer_output.flatten(2)
hidden_layer_input = flatten_layer_output
hidden_layer_size = input_shape[1] * input_shape[2] * input_shape[3]
for hidden_size in hidden_sizes:
W = theano.shared(
np.asarray(
rng.uniform(low=-np.sqrt(6. / (hidden_layer_size + hidden_size)), high=np.sqrt(6. / (hidden_layer_size + hidden_size)), size=(hidden_layer_size, hidden_size)),
dtype=theano.config.floatX
),
borrow=True
)
b = theano.shared(
np.zeros((hidden_size,), dtype=theano.config.floatX),
borrow=True
)
self.params += [W, b]
hidden_layer_output = T.nnet.sigmoid(T.dot(hidden_layer_input, W) + b)
hidden_layer_input = hidden_layer_output
hidden_layer_size = hidden_size
W = theano.shared(
np.asarray(
rng.uniform(low=-np.sqrt(6. / (hidden_layer_size + output_size)), high=np.sqrt(6. / (hidden_layer_size + output_size)), size=(hidden_layer_size, output_size)),
dtype=theano.config.floatX
),
borrow=True
)
b = theano.shared(
np.zeros((output_size,), dtype=theano.config.floatX),
borrow=True
)
self.params += [W, b]
self.output = T.nnet.softmax(T.dot(hidden_layer_output, W) + b)
self.prediction = T.argmax(self.output, axis=1)
self.cost = -T.mean(T.log(self.output)[T.arange(self.y.shape[0]), self.y])
self.updates = self.layers[0].get_cost_updates(self.cost, learning_rate=0.1)
for i in range(1, len(self.layers)):
layer_updates = self.layers[i].get_cost_updates(self.cost, learning_rate=0.1)
self.updates += layer_updates
self.train = theano.function(inputs=[self.x, self.y], outputs=self.cost, updates=self.updates, allow_input_downcast=True)
self.predict = theano.function(inputs=[self.x], outputs=self.prediction, allow_input_downcast=True)
```
最后,我们可以使用以下代码来训练和测试模型。
```python
rng = np.random.RandomState(1234)
train_x = np.random.rand(100, 1, 28, 28).astype(theano.config.floatX)
train_y = np.random.randint(0, 10, size=(100,)).astype(np.int32)
mlp = MLP(rng=rng, input_shape=(100, 1, 28, 28), filter_shapes=[(20, 1, 5, 5), (50, 20, 5, 5)], hidden_sizes=[500], output_size=10)
for i in range(10):
cost = mlp.train(train_x, train_y)
print('Epoch %d, cost %f' % (i, cost))
test_x = np.random.rand(10, 1, 28, 28).astype(theano.config.floatX)
test_y = np.random.randint(0, 10, size=(10,)).astype(np.int32)
pred_y = mlp.predict(test_x)
accuracy = np.mean(pred_y == test_y)
print('Accuracy %f' % accuracy)
```
阅读全文
相关推荐
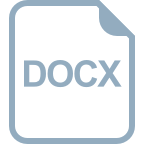
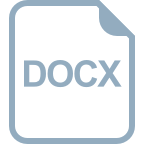
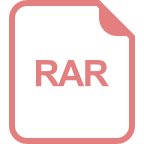
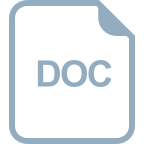
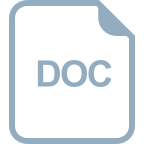
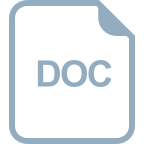
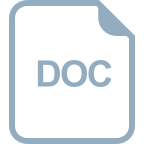
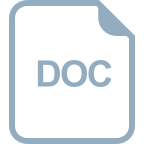
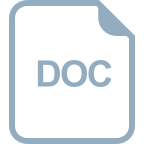
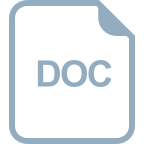
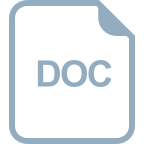
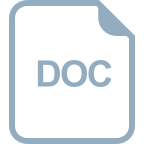
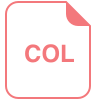
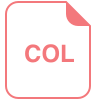
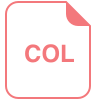