c++怎么读取csv文件
时间: 2023-10-08 18:13:46 浏览: 149
您可以使用Python中的`csv`模块来读取CSV文件。
以下是一个简单的示例代码,展示了如何读取CSV文件并打印出其中的数据:
```python
import csv
# 打开CSV文件
with open('file.csv', 'r') as file:
# 创建CSV读取器
reader = csv.reader(file)
# 遍历每一行数据
for row in reader:
# 打印行数据
print(row)
```
在这个示例中,我们使用`open`函数打开名为`file.csv`的CSV文件,并使用`csv.reader`创建一个CSV读取器。然后,我们可以通过遍历读取器来逐行访问数据,并使用`print`语句打印出每一行数据。
请确保将代码中的`file.csv`替换为您实际的CSV文件路径。
相关问题
C++ 读取CSV文件
你可以使用 C++ 标准库中的 `<fstream>` 头文件中的 `ifstream` 类来读取CSV文件,然后使用字符串分割函数对行进行分割,再使用字符串转数字函数将数据转换为具体数据类型。具体代码如下所示:
```c++
#include <fstream>
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
std::vector<std::vector<double>> readCSV(const std::string& filePath, char delimiter = ',')
{
std::vector<std::vector<double>> data;
std::ifstream file(filePath);
if (!file.is_open())
{
std::cout << "Failed to open file: " << filePath << std::endl;
return data;
}
std::string line;
while (std::getline(file, line))
{
std::vector<double> row;
std::stringstream ss(line);
std::string cell;
while (std::getline(ss, cell, delimiter))
{
row.push_back(std::stod(cell));
}
data.push_back(row);
}
return data;
}
```
上述代码中,`readCSV` 函数将读取指定 CSV 文件,将数据存储在一个二维数组中,并返回该数组。其中,`filePath` 是指定的 CSV 文件路径,`delimiter` 是 CSV 文件的分隔符。在函数中,先判断文件是否成功打开,然后逐行读取 CSV 文件,使用 `std::stringstream` 对每行进行分割,再将分割后的每个字符串转换为 `double` 类型,并将其存储在一个二维数组中。最后,将该数组返回。
C++读取csv文件
要读取CSV文件,你可以使用C++中的ifstream类来实现。首先,你需要包含头文件<fstream>。然后,你可以创建一个ifstream对象,并打开CSV文件。接下来,你可以使用getline函数来逐行读取文件内容,并将每行内容存储在一个字符串中。你可以使用逗号作为分隔符来分割每行的数据,并将它们存储在一个数组或向量中。最后,你可以关闭文件并处理读取到的数据。
以下是一个示例代码来展示如何读取CSV文件:
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
int main() {
ifstream inFile("Data.csv"); // 打开CSV文件
if (!inFile) {
cout << "打开文件失败!" << endl;
exit(1);
}
string line;
vector<vector<string>> data; // 存储读取到的数据
while (getline(inFile, line)) { // 逐行读取文件内容
vector<string> row;
string cell;
stringstream lineStream(line);
while (getline(lineStream, cell, ',')) { // 逗号作为分隔符分割每行的数据
row.push_back(cell);
}
data.push_back(row);
}
inFile.close(); // 关闭文件
// 处理读取到的数据
for (int i = 0; i < data.size(); i++) {
for (int j = 0; j < data[i].size(); j++) {
cout << data[i][j] << " ";
}
cout << endl;
}
cout << "读取数据完成" << endl;
return 0;
}
阅读全文
相关推荐




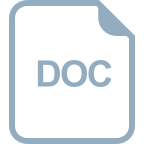










