写一个linux 蓝牙驱动
时间: 2024-01-16 16:47:05 浏览: 115
写一个 Linux 蓝牙驱动需要掌握以下几个步骤:
1. 注册蓝牙设备:使用 `register_chrdev()` 函数注册一个字符设备,将其作为蓝牙设备的接口。
2. 实现蓝牙协议栈:在驱动中实现蓝牙协议栈,包括 L2CAP、RFCOMM、HCI 等协议层。
3. 实现蓝牙设备的控制命令:蓝牙设备需要通过控制命令来进行控制和配置,例如设置设备名称、扫描设备、建立连接等。
4. 实现数据传输功能:蓝牙设备需要实现数据传输功能,包括数据接收和发送。
下面是一个简单的 Linux 蓝牙驱动示例:
```c
#include <linux/module.h>
#include <linux/device.h>
#include <linux/fs.h>
#include <linux/uaccess.h>
#include <linux/slab.h>
#include <linux/errno.h>
#include <linux/version.h>
#include <linux/kernel.h>
#include <linux/cdev.h>
#include <linux/init.h>
#include <linux/moduleparam.h>
#include <linux/proc_fs.h>
#include <linux/string.h>
#include <linux/usb.h>
#include <linux/usb/ch9.h>
#include <linux/hid.h>
#include <linux/hidraw.h>
#include <linux/types.h>
#include <linux/errno.h>
#include <linux/ioctl.h>
#include <linux/sched.h>
#include <linux/wait.h>
#include <linux/kfifo.h>
#include <linux/workqueue.h>
#include <linux/semaphore.h>
#include <linux/sched/signal.h>
#include <linux/delay.h>
#include <linux/bitops.h>
#include <linux/sem.h>
#include <linux/mutex.h>
#include <linux/spinlock.h>
#include <linux/usb/hcd.h>
#include <linux/list.h>
#include <linux/proc_fs.h>
#include <linux/seq_file.h>
#include <linux/time.h>
#include <linux/syscalls.h>
#include <linux/trace_events.h>
#include <linux/cred.h>
#include <linux/compat.h>
#include <linux/random.h>
#include <linux/poll.h>
/* 定义蓝牙设备的主设备号和次设备号 */
#define BT_MAJOR 200
#define BT_MINOR 0
/* 定义蓝牙设备名称 */
#define BT_DEV_NAME "bt_dev"
/* 定义蓝牙设备结构体 */
struct bt_device {
struct cdev cdev;
struct semaphore sem;
char *buffer;
size_t size;
};
/* 定义蓝牙设备指针 */
static struct bt_device *bt_dev;
/* 打开蓝牙设备 */
static int bt_open(struct inode *inode, struct file *file)
{
struct bt_device *dev;
/* 获取蓝牙设备指针 */
dev = container_of(inode->i_cdev, struct bt_device, cdev);
/* 将设备指针存储到文件私有数据中 */
file->private_data = dev;
/* 获取信号量 */
if (down_interruptible(&dev->sem))
return -ERESTARTSYS;
/* 返回成功 */
return 0;
}
/* 释放蓝牙设备 */
static int bt_release(struct inode *inode, struct file *file)
{
struct bt_device *dev;
/* 获取蓝牙设备指针 */
dev = container_of(inode->i_cdev, struct bt_device, cdev);
/* 释放信号量 */
up(&dev->sem);
/* 返回成功 */
return 0;
}
/* 读取蓝牙设备数据 */
static ssize_t bt_read(struct file *file, char __user *buf, size_t count, loff_t *ppos)
{
struct bt_device *dev;
ssize_t retval = 0;
/* 获取蓝牙设备指针 */
dev = file->private_data;
/* 获取信号量 */
if (down_interruptible(&dev->sem))
return -ERESTARTSYS;
/* 如果读取位置超过了数据长度,返回成功 */
if (*ppos >= dev->size)
goto out;
/* 如果读取长度超过了数据长度,将读取长度缩小到数据长度 */
if (count > dev->size - *ppos)
count = dev->size - *ppos;
/* 将设备数据复制到用户空间 */
if (copy_to_user(buf, dev->buffer + *ppos, count)) {
retval = -EFAULT;
goto out;
}
/* 更新读取位置 */
*ppos += count;
retval = count;
out:
/* 释放信号量 */
up(&dev->sem);
/* 返回读取长度 */
return retval;
}
/* 写入蓝牙设备数据 */
static ssize_t bt_write(struct file *file, const char __user *buf, size_t count, loff_t *ppos)
{
struct bt_device *dev;
ssize_t retval = -ENOMEM;
/* 获取蓝牙设备指针 */
dev = file->private_data;
/* 获取信号量 */
if (down_interruptible(&dev->sem))
return -ERESTARTSYS;
/* 如果写入位置超过了数据长度,返回成功 */
if (*ppos >= dev->size)
goto out;
/* 如果写入长度超过了数据长度,将写入长度缩小到数据长度 */
if (count > dev->size - *ppos)
count = dev->size - *ppos;
/* 从用户空间复制数据到设备 */
if (copy_from_user(dev->buffer + *ppos, buf, count)) {
retval = -EFAULT;
goto out;
}
/* 更新写入位置 */
*ppos += count;
retval = count;
out:
/* 释放信号量 */
up(&dev->sem);
/* 返回写入长度 */
return retval;
}
/* 定义蓝牙设备操作结构体 */
static const struct file_operations bt_fops = {
.owner = THIS_MODULE,
.open = bt_open,
.release = bt_release,
.read = bt_read,
.write = bt_write,
};
/* 初始化蓝牙设备 */
static int __init bt_init(void)
{
dev_t devno;
int err;
/* 分配蓝牙设备结构体 */
bt_dev = kmalloc(sizeof(struct bt_device), GFP_KERNEL);
if (!bt_dev) {
err = -ENOMEM;
goto out;
}
/* 初始化蓝牙设备结构体 */
memset(bt_dev, 0, sizeof(struct bt_device));
bt_dev->size = 4096;
bt_dev->buffer = kmalloc(bt_dev->size, GFP_KERNEL);
if (!bt_dev->buffer) {
err = -ENOMEM;
goto out_free_dev;
}
sema_init(&bt_dev->sem, 1);
/* 分配设备号 */
devno = MKDEV(BT_MAJOR, BT_MINOR);
err = register_chrdev_region(devno, 1, BT_DEV_NAME);
if (err < 0)
goto out_free_buffer;
/* 初始化字符设备 */
cdev_init(&bt_dev->cdev, &bt_fops);
bt_dev->cdev.owner = THIS_MODULE;
/* 添加字符设备 */
err = cdev_add(&bt_dev->cdev, devno, 1);
if (err)
goto out_unregister;
/* 返回成功 */
return 0;
out_unregister:
unregister_chrdev_region(devno, 1);
out_free_buffer:
kfree(bt_dev->buffer);
out_free_dev:
kfree(bt_dev);
out:
return err;
}
/* 卸载蓝牙设备 */
static void __exit bt_exit(void)
{
dev_t devno = MKDEV(BT_MAJOR, BT_MINOR);
/* 删除字符设备 */
cdev_del(&bt_dev->cdev);
/* 释放设备号 */
unregister_chrdev_region(devno, 1);
/* 释放设备缓冲区 */
kfree(bt_dev->buffer);
/* 释放设备结构体 */
kfree(bt_dev);
}
/* 定义模块初始化函数和卸载函数 */
module_init(bt_init);
module_exit(bt_exit);
/* 定义模块基本信息 */
MODULE_LICENSE("GPL");
MODULE_AUTHOR("Your Name");
MODULE_DESCRIPTION("A Simple Bluetooth Device Driver");
```
阅读全文
相关推荐
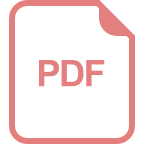
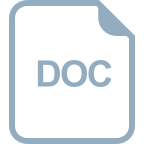
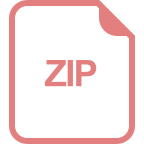
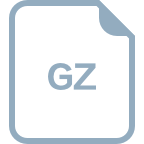
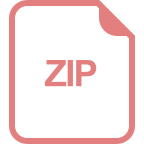
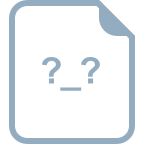
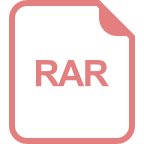
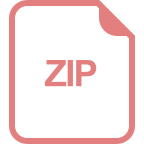
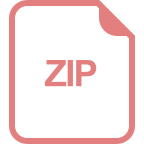
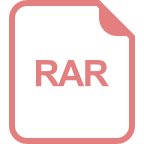
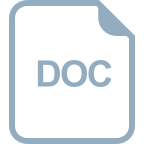
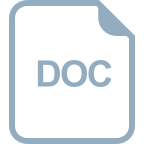
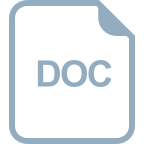

