C# 设置 icon为按钮图标
时间: 2023-10-06 09:10:54 浏览: 57
在C#中,你可以将一个icon设置为按钮的图标,具体实现方法如下:
1. 从资源中加载icon文件
在Visual Studio中,你可以将icon文件添加到项目资源中。在资源管理器中,右击“资源文件”文件夹,选择“添加现有项”,然后选择要添加的icon文件。添加后,你可以使用下面的代码从资源中加载icon文件:
```csharp
Icon icon = Properties.Resources.iconName; // 将iconName替换成实际的资源文件名
```
2. 将icon设置为按钮的图标
在窗体的Load事件中,你可以使用下面的代码将icon设置为按钮的图标:
```csharp
private void Form1_Load(object sender, EventArgs e)
{
Icon icon = Properties.Resources.iconName; // 将iconName替换成实际的资源文件名
button1.Icon = icon.ToBitmap();
}
```
其中,button1是你要设置图标的按钮控件。通过调用icon的ToBitmap()方法,将icon转换为Bitmap格式,然后将其设置为按钮的图标。
希望这可以帮助到你。
相关问题
C#怎么在DataGridViewButtonCell按钮中添加图标,有例子吗?
可以通过自定义DataGridViewButtonCell来实现在按钮中添加图标。
以下是一个示例代码,其中使用了一个名为“icon”的Image变量,它将作为图标显示在按钮上:
```
public class DataGridViewIconButtonCell : DataGridViewButtonCell
{
private Image icon;
public Image Icon
{
get { return icon; }
set { icon = value; }
}
protected override void Paint(Graphics graphics, Rectangle clipBounds, Rectangle cellBounds, int rowIndex, DataGridViewElementStates elementState, object value, object formattedValue, string errorText, DataGridViewCellStyle cellStyle, DataGridViewAdvancedBorderStyle advancedBorderStyle, DataGridViewPaintParts paintParts)
{
base.Paint(graphics, clipBounds, cellBounds, rowIndex, elementState, value, formattedValue, errorText, cellStyle, advancedBorderStyle, paintParts);
if (icon != null)
{
// 绘制图标
graphics.DrawImage(icon, cellBounds.Left + 4, cellBounds.Top + 4, 16, 16);
}
}
}
```
然后,您可以将此自定义单元格类型应用于DataGridView中的单元格,例如:
```
// 创建一个新的自定义按钮单元格
DataGridViewIconButtonCell cell = new DataGridViewIconButtonCell();
cell.Icon = Properties.Resources.icon; // 设置图标
// 将单元格添加到DataGridView中的某一行
dataGridView1.Rows[0].Cells[0] = cell;
```
在上面的示例中,我们将单元格中的图标设置为一个名为“icon”的资源文件。您需要将其替换为您自己的图标。
C#winform窗口图标
要在 C# WinForm 窗口中设置图标,可以使用以下代码:
```csharp
this.Icon = new Icon("icon.ico");
```
其中,"icon.ico" 是你想要设置的图标文件路径。你也可以直接使用 Visual Studio 中的窗口属性设置窗口图标。在窗口的属性窗口中,找到 "Icon" 属性,点击 "..." 按钮选择你想要设置的图标文件即可。
相关推荐
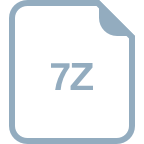












