使用C++语言,编写一段程序,从标准输入中一次读入一整行,存入std::string 中,然后修改该程序,使其一次读入一个词。
时间: 2023-05-11 13:02:56 浏览: 103
以下是使用C语言编写的程序,可以从标准输入中一次读入一整行,存入std::string中:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 1024
int main() {
char line[MAX_LEN];
fgets(line, MAX_LEN, stdin);
line[strcspn(line, "\n")] = '\0'; // remove trailing newline
printf("Input line: %s\n", line);
return 0;
}
```
为了使其一次读入一个词,我们可以使用scanf函数,如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 1024
int main() {
char word[MAX_LEN];
scanf("%s", word);
printf("Input word: %s\n", word);
return 0;
}
```
注意,这个程序只能读取一个单词,如果输入的是一个带空格的句子,它只会读取第一个单词。如果需要读取整个句子,可以使用fgets函数,如上所示。
相关问题
编写一段程序,从标准输入中一次读入一整行,存入 std::string 中,然后 修改该程序,使其一次读入一个词。用c++实现
读取一整行并存入 std::string 的程序:
```cpp
#include <iostream>
#include <string>
int main() {
std::string line;
std::getline(std::cin, line);
std::cout << "Input line: " << line << std::endl;
return 0;
}
```
一次读入一个词的程序:
```cpp
#include <iostream>
#include <string>
int main() {
std::string word;
std::cin >> word;
std::cout << "Input word: " << word << std::endl;
return 0;
}
```
注意,std::cin 默认以空格作为分隔符,因此在输入时需要以空格为分隔符输入每个词。如果需要读取整个行中的一个词,可以使用 std::getline() 读取整行并再次分割。
编写一段程序,从标准输入中一次读入一整行,存入 std::string 中,然后 修改该程序,使其一次读入一个词。
读入一整行的程序:
```c++
#include <iostream>
#include <string>
int main() {
std::string line;
std::getline(std::cin, line);
std::cout << "You entered: " << line << std::endl;
return 0;
}
```
读入一个词的程序:
```c++
#include <iostream>
#include <string>
int main() {
std::string word;
std::cin >> word;
std::cout << "You entered: " << word << std::endl;
return 0;
}
```
这里使用了`std::cin`输入流,它默认以空格为分隔符,遇到空格就会停止读取。如果需要读取整个行,可以使用`std::getline`函数,它可以读取一整行,直到遇到换行符为止。
阅读全文
相关推荐
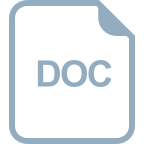
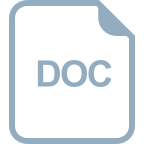
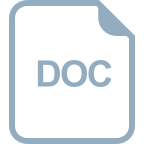


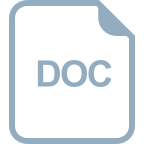










