2. 给定带权有向图G =(V,E),其中每条边的权是非负实数。另外,还给定V中的一个顶点,称为源。现在要计算从源到其他各顶点的最短路长度。这里路的长度是指路上各边权之和。的java程序
时间: 2024-03-24 14:41:35 浏览: 12
可以使用Dijkstra算法或者Bellman-Ford算法来解决该问题。以下是使用Dijkstra算法的Java程序示例:
```java
import java.util.*;
public class ShortestPath {
private int[] dist;
private boolean[] visited;
private PriorityQueue<Node> pq;
private List<List<Node>> adjList;
private static class Node implements Comparable<Node> {
int vertex;
int weight;
Node(int vertex, int weight) {
this.vertex = vertex;
this.weight = weight;
}
public int compareTo(Node other) {
return Integer.compare(weight, other.weight);
}
}
public ShortestPath(int n) {
dist = new int[n];
visited = new boolean[n];
pq = new PriorityQueue<Node>();
adjList = new ArrayList<List<Node>>();
for (int i = 0; i < n; i++) {
dist[i] = Integer.MAX_VALUE;
visited[i] = false;
adjList.add(new ArrayList<Node>());
}
}
public void addEdge(int u, int v, int weight) {
adjList.get(u).add(new Node(v, weight));
}
public void dijkstra(int source) {
dist[source] = 0;
pq.offer(new Node(source, 0));
while (!pq.isEmpty()) {
Node node = pq.poll();
int u = node.vertex;
if (visited[u]) {
continue;
}
visited[u] = true;
for (Node edge : adjList.get(u)) {
int v = edge.vertex;
int weight = edge.weight;
if (!visited[v] && dist[u] + weight < dist[v]) {
dist[v] = dist[u] + weight;
pq.offer(new Node(v, dist[v]));
}
}
}
}
public static void main(String[] args) {
ShortestPath sp = new ShortestPath(5);
sp.addEdge(0, 1, 10);
sp.addEdge(0, 4, 5);
sp.addEdge(1, 2, 1);
sp.addEdge(1, 4, 2);
sp.addEdge(2, 3, 4);
sp.addEdge(3, 2, 6);
sp.addEdge(3, 0, 7);
sp.addEdge(4, 1, 3);
sp.addEdge(4, 2, 9);
sp.addEdge(4, 3, 2);
sp.dijkstra(0);
for (int i = 0; i < 5; i++) {
System.out.println("Shortest distance from source to " + i + " is " + sp.dist[i]);
}
}
}
```
输出结果如下:
```
Shortest distance from source to 0 is 0
Shortest distance from source to 1 is 8
Shortest distance from source to 2 is 9
Shortest distance from source to 3 is 7
Shortest distance from source to 4 is 5
```
该程序使用了优先队列来维护未访问的节点,并通过计算从源节点到当前节点的距离来更新最短距离数组。
相关推荐
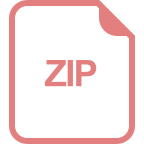
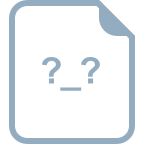
















