一个简单的多线程文件拷贝程序,包含一个主线程和多个工作线程。主线程从命令行参数中获取源文件和目标文件名,然后将源文件分成若干块并分配给工作线程进行并行拷贝。每个工作线程负责拷贝一块数据,并将其写入目标文件中。使用线程间通信和线程同步技术来避免竞态条件。java实现
时间: 2024-05-13 12:15:55 浏览: 25
以下是一个简单的多线程文件拷贝程序的示例代码,其中包含一个主线程和多个工作线程。在这个示例中,我们使用了Java的线程池来管理工作线程,并使用阻塞队列实现线程间通信和线程同步。请注意,这只是一个示例,实际应用中可能需要根据具体需求进行修改和优化。
```java
import java.io.*;
import java.util.concurrent.*;
public class FileCopy {
public static void main(String[] args) {
if (args.length != 2) {
System.err.println("Usage: FileCopy <source file> <target file>");
System.exit(1);
}
String sourceFile = args[0];
String targetFile = args[1];
int numThreads = Runtime.getRuntime().availableProcessors();
try {
// 创建输入输出流
FileInputStream fis = new FileInputStream(sourceFile);
FileOutputStream fos = new FileOutputStream(targetFile);
// 创建阻塞队列
BlockingQueue<byte[]> queue = new LinkedBlockingQueue<>();
// 创建线程池
ExecutorService executor = Executors.newFixedThreadPool(numThreads);
// 启动工作线程
for (int i = 0; i < numThreads; i++) {
executor.execute(new WorkerThread(i, fis, fos, queue));
}
// 读取源文件并将数据块放入队列
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = fis.read(buffer)) != -1) {
byte[] block = new byte[bytesRead];
System.arraycopy(buffer, 0, block, 0, bytesRead);
queue.put(block);
}
// 发送结束信号
for (int i = 0; i < numThreads; i++) {
queue.put(new byte[0]);
}
// 等待所有工作线程完成
executor.shutdown();
executor.awaitTermination(Long.MAX_VALUE, TimeUnit.NANOSECONDS);
// 关闭输入输出流
fis.close();
fos.close();
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
static class WorkerThread implements Runnable {
private final int id;
private final FileInputStream fis;
private final FileOutputStream fos;
private final BlockingQueue<byte[]> queue;
public WorkerThread(int id, FileInputStream fis, FileOutputStream fos, BlockingQueue<byte[]> queue) {
this.id = id;
this.fis = fis;
this.fos = fos;
this.queue = queue;
}
public void run() {
try {
while (true) {
byte[] block = queue.take();
if (block.length == 0) {
// 收到结束信号,退出循环
break;
}
// 拷贝数据块
fos.getChannel().write(ByteBuffer.wrap(block));
System.out.println("Thread " + id + ": copied " + block.length + " bytes");
}
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
相关推荐
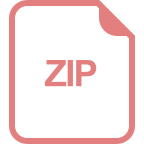
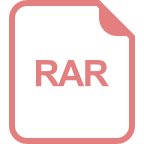
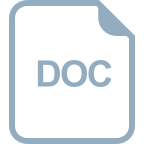
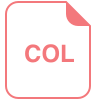
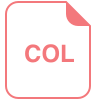
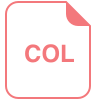
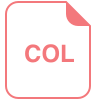
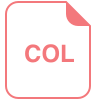









