在Vue 3和Vuex中 根据路径对对象的增删改
时间: 2024-04-09 19:27:31 浏览: 173
在Vue 3和Vuex中,可以使用Vue的响应式系统和Vue.set/Vue.delete函数来根据路径对对象进行增删改操作。以下是一个示例:
首先,在Vuex的mutations中定义相应的函数来处理对象的增删改操作:
```javascript
// 在mutations中定义一个函数用于增加对象属性
addProperty(state, payload) {
const pathArray = payload.path.split('.');
let target = state.object;
for (let i = 0; i < pathArray.length - 1; i++) {
const key = pathArray[i];
if (!target[key]) {
Vue.set(target, key, {});
}
target = target[key];
}
const lastKey = pathArray[pathArray.length - 1];
Vue.set(target, lastKey, payload.value);
}
// 在mutations中定义一个函数用于删除对象属性
deleteProperty(state, path) {
const pathArray = path.split('.');
let target = state.object;
for (let i = 0; i < pathArray.length - 1; i++) {
const key = pathArray[i];
if (!target[key]) {
return;
}
target = target[key];
}
const lastKey = pathArray[pathArray.length - 1];
Vue.delete(target, lastKey);
}
// 在mutations中定义一个函数用于修改对象属性
updateProperty(state, payload) {
const pathArray = payload.path.split('.');
let target = state.object;
for (let i = 0; i < pathArray.length - 1; i++) {
const key = pathArray[i];
if (!target[key]) {
return;
}
target = target[key];
}
const lastKey = pathArray[pathArray.length - 1];
Vue.set(target, lastKey, payload.value);
}
```
然后,在组件中可以通过调用this.$store.commit来触发对应的mutation函数,并传递相应的payload对象或路径作为参数:
```javascript
// 增加对象属性
this.$store.commit('addProperty', { path: 'object.property', value: 'new value' });
// 删除对象属性
this.$store.commit('deleteProperty', 'object.property');
// 修改对象属性
this.$store.commit('updateProperty', { path: 'object.property', value: 'updated value' });
```
这样就可以根据路径对对象进行增删改操作了,使用Vue.set和Vue.delete方法可以确保响应式更新。记得在mutations中使用这两个方法来操作对象属性。
阅读全文
相关推荐
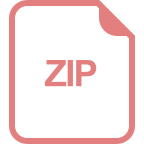
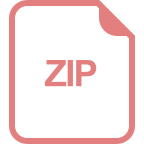

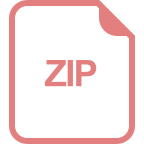
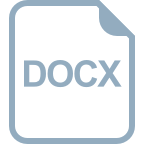
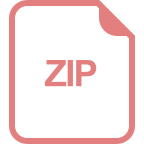
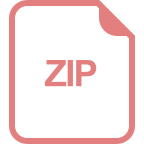
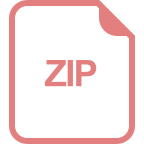
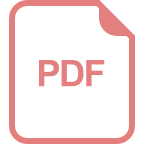
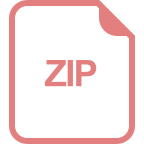
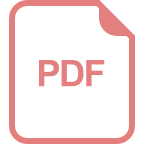
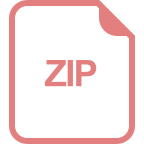
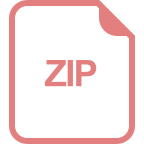
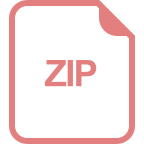
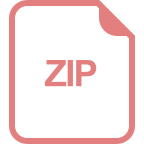
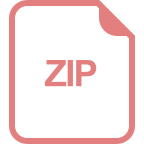
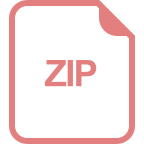