借助词法分析生成器Lex和语法分析生成器Yacc完成一个简单的Linux命令解释器
时间: 2024-01-24 19:16:56 浏览: 100
好的,我可以给你提供一个简单的Linux命令解释器的实现思路,使用Lex和Yacc完成。具体步骤如下:
1. 定义命令的语法规则:可以定义一些简单的命令,例如cd、ls、mkdir等。
2. 使用Lex编写词法分析器:根据语法规则,将输入的命令字符串分解为多个token,方便后续的语法分析。
3. 使用Yacc编写语法分析器:根据语法规则,对输入的token进行语法分析,生成语法树,并执行相应的操作。
4. 实现命令的操作:根据具体的命令需求,实现对应的操作函数,例如cd的路径更改、ls的文件列表输出等。
下面是一个简单的例子:
1. 定义命令的语法规则
```
command : cd_command
| ls_command
| mkdir_command
;
cd_command : CD PATH
;
ls_command : LS
;
mkdir_command : MKDIR PATH
;
```
2. 使用Lex编写词法分析器
```
%{
#include "y.tab.h"
%}
%%
cd { return CD; }
ls { return LS; }
mkdir { return MKDIR; }
[ \t\n] { /* ignore whitespace */ }
. { printf("Invalid character %c\n", *yytext); }
%%
int yywrap(void) {
return 1;
}
```
3. 使用Yacc编写语法分析器
```
%{
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
%}
%token CD LS MKDIR PATH
%%
command : cd_command
| ls_command
| mkdir_command
;
cd_command : CD PATH { chdir($2); }
;
ls_command : LS { system("ls"); }
;
mkdir_command : MKDIR PATH { mkdir($2, 0777); }
;
%%
int main(void) {
yyparse();
return 0;
}
void yyerror(char *msg) {
printf("%s\n", msg);
}
```
4. 实现命令的操作
在上面的语法分析器中,我们已经定义了相应的操作函数。具体实现可以参考对应命令的系统调用或者库函数。
以上是一个简单的Linux命令解释器的实现思路,希望对你有所帮助!
阅读全文
相关推荐






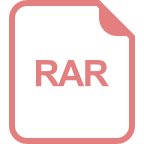










