用代码修改Android 输入法
时间: 2023-12-11 07:02:49 浏览: 31
要修改 Android 输入法,可以通过继承 InputMethodService 实现自己的输入法,并在 Android 系统中注册使用。以下是一个简单的示例代码,可以在 `onCreateInputView()` 方法中添加自定义的输入法界面:
```java
public class MyInputMethodService extends InputMethodService {
@Override
public View onCreateInputView() {
// 创建自定义的输入法界面,例如一个包含数字和字母的键盘
LayoutInflater inflater = (LayoutInflater) getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View keyboardView = inflater.inflate(R.layout.keyboard_layout, null);
// 为键盘上的按键设置点击事件,例如在点击数字键时向输入框中插入相应的数字
keyboardView.findViewById(R.id.button0).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
InputConnection inputConnection = getCurrentInputConnection();
if (inputConnection != null) {
inputConnection.commitText("0", 1);
}
}
});
// 返回自定义的输入法界面
return keyboardView;
}
}
```
在 AndroidManifest.xml 文件中注册该输入法:
```xml
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myinputmethod">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme">
<service
android:name=".MyInputMethodService"
android:label="@string/app_name"
android:permission="android.permission.BIND_INPUT_METHOD">
<meta-data
android:name="android.view.im"
android:resource="@xml/method" />
<intent-filter>
<action android:name="android.view.InputMethod" />
</intent-filter>
</service>
</application>
</manifest>
```
其中,`@xml/method` 文件定义了输入法的配置信息:
```xml
<?xml version="1.0" encoding="utf-8"?>
<input-method xmlns:android="http://schemas.android.com/apk/res/android"
android:settingsActivity="com.example.myinputmethod.SettingsActivity"
android:canRetrieveWindowToken="true"
android:isDefault="true" />
```
在设置界面中,可以通过 `android:settingsActivity` 指定设置界面的 Activity,通过 `android:isDefault` 指定该输入法是否为默认输入法。
相关推荐
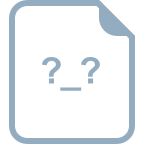
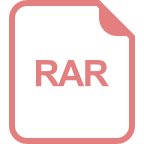
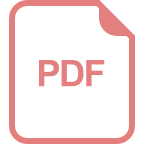














