使用定时器实现 函数的超时功能
时间: 2023-12-26 10:03:47 浏览: 147
在C++中,可以使用定时器来实现函数的超时功能。主要思路是在一个新线程中启动定时器,并在规定时间内检测函数是否执行完毕,若未执行完毕则强制结束函数执行。具体实现步骤如下:
1. 定义超时信号
在Linux环境下,可以使用SIGALRM信号实现超时功能。在Windows环境下,则需要使用CreateTimerQueueTimer创建定时器。
2. 创建新线程
使用std::thread创建一个新线程,在新线程中启动定时器,并等待函数执行完毕。
3. 执行函数
在主线程中执行函数。
4. 判断超时
在新线程中检测函数是否执行完毕,若未执行完毕则强制结束函数执行。
示例代码如下:
```c++
#include <iostream>
#include <thread>
#include <chrono>
#include <csignal>
using namespace std;
void timeoutHandler(int sig)
{
cout << "Function timeout!" << endl;
exit(1);
}
void testFunction()
{
// 执行代码
this_thread::sleep_for(chrono::seconds(3));
}
void runWithTimeout(int timeout, function<void()> func)
{
signal(SIGALRM, timeoutHandler); // 注册超时信号
alarm(timeout); // 启动定时器
func(); // 执行函数
alarm(0); // 取消定时器
}
int main()
{
thread timeoutThread(runWithTimeout, 2, testFunction); // 创建新线程
timeoutThread.join(); // 等待线程结束
return 0;
}
```
以上代码实现了一个在Linux环境下的函数超时功能。在Windows环境下,需要使用CreateTimerQueueTimer创建定时器,具体实现可以参考Windows API文档。
阅读全文
相关推荐






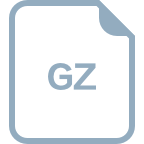










