可以写一个四叉树代码吗
时间: 2023-07-16 18:14:59 浏览: 68
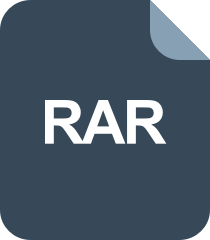
四叉树代码
当然可以,下面是一个简单的四叉树实现代码:
```
class QuadTree:
def __init__(self, x1, y1, x2, y2, capacity=4):
self.x1 = x1
self.y1 = y1
self.x2 = x2
self.y2 = y2
self.capacity = capacity
self.points = []
self.northwest = None
self.northeast = None
self.southwest = None
self.southeast = None
def insert(self, point):
if len(self.points) < self.capacity:
self.points.append(point)
else:
if self.northwest is None:
self.subdivide()
if self.northwest.contains(point):
self.northwest.insert(point)
elif self.northeast.contains(point):
self.northeast.insert(point)
elif self.southwest.contains(point):
self.southwest.insert(point)
else:
self.southeast.insert(point)
def subdivide(self):
x_mid = (self.x1 + self.x2) / 2
y_mid = (self.y1 + self.y2) / 2
self.northwest = QuadTree(self.x1, self.y1, x_mid, y_mid, self.capacity)
self.northeast = QuadTree(x_mid, self.y1, self.x2, y_mid, self.capacity)
self.southwest = QuadTree(self.x1, y_mid, x_mid, self.y2, self.capacity)
self.southeast = QuadTree(x_mid, y_mid, self.x2, self.y2, self.capacity)
def contains(self, point):
return self.x1 <= point[0] <= self.x2 and self.y1 <= point[1] <= self.y2
def query(self, range):
points_within_range = []
if not self.intersects(range):
return points_within_range
for point in self.points:
if range.contains(point):
points_within_range.append(point)
if self.northwest is None:
return points_within_range
points_within_range.extend(self.northwest.query(range))
points_within_range.extend(self.northeast.query(range))
points_within_range.extend(self.southwest.query(range))
points_within_range.extend(self.southeast.query(range))
return points_within_range
def intersects(self, range):
return not (self.x1 > range.x2 or self.x2 < range.x1 or self.y1 > range.y2 or self.y2 < range.y1)
```
这个四叉树类有以下方法:
- `__init__(self, x1, y1, x2, y2, capacity=4)`:初始化一个四叉树,x1, y1, x2, y2 是四叉树的边界,capacity 是每个节点最多容纳的点的数量。
- `insert(self, point)`:将一个点插入四叉树中。
- `subdivide(self)`:将节点分成四个子节点。
- `contains(self, point)`:判断一个点是否在当前节点内。
- `query(self, range)`:查询一个范围内的所有点,range 是一个矩形,表示要查询的范围。
- `intersects(self, range)`:判断一个范围是否与当前节点相交。
阅读全文
相关推荐
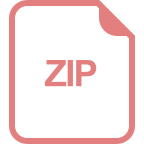
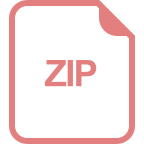
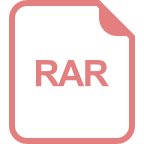
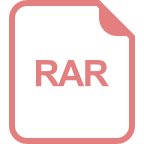
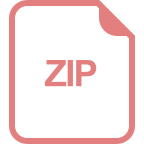
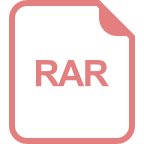
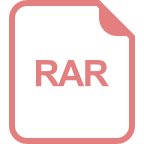
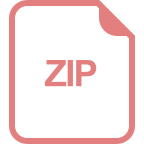
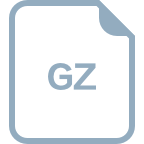
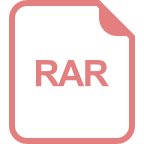
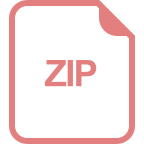
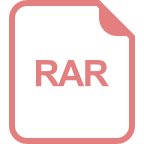
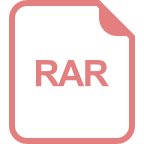
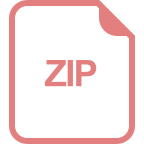