use Java to achieve that:Write a program to display font sizes Layout It should have: ● In the top: - label for letter “X” - label for font size value ● In the bottom: - button to increase font size - button to decrease font size Desired final look: Functionality Initially, the X label should display an 18 point “X”, and the font size label should display the value “18”. Every time the “increase” button is selected, the font size of the “X” and the value in the font size label should be increased by 1. Every time the “decrease” button is selected, the font size of the “X” and the value in the font size label should be decreased by 1. Make sure that this does not allow negative font sizes.
时间: 2024-01-15 07:03:15 浏览: 62
Sure, here is the Java code to achieve the same functionality:
```java
import java.awt.Font;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class FontSizer extends JFrame {
private JLabel xLabel;
private JLabel fontSizeLabel;
private JButton increaseButton;
private JButton decreaseButton;
private int fontSize;
public FontSizer() {
super("Font Sizer");
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setSize(300, 200);
// create top panel with "X" label and font size label
JPanel topPanel = new JPanel();
this.xLabel = new JLabel("X");
this.fontSize = 18;
this.fontSizeLabel = new JLabel(Integer.toString(this.fontSize));
this.fontSizeLabel.setFont(new Font("Helvetica", Font.PLAIN, 14));
topPanel.add(this.xLabel);
topPanel.add(this.fontSizeLabel);
// create bottom panel with increase and decrease buttons
JPanel bottomPanel = new JPanel();
this.increaseButton = new JButton("Increase Font Size");
this.increaseButton.addActionListener(e -> increaseFontSize());
this.decreaseButton = new JButton("Decrease Font Size");
this.decreaseButton.addActionListener(e -> decreaseFontSize());
bottomPanel.add(this.increaseButton);
bottomPanel.add(this.decreaseButton);
// add panels to main frame
this.add(topPanel);
this.add(bottomPanel, "South");
this.setVisible(true);
}
private void increaseFontSize() {
this.fontSize++;
updateFontSize();
}
private void decreaseFontSize() {
if (this.fontSize > 1) {
this.fontSize--;
updateFontSize();
}
}
private void updateFontSize() {
this.xLabel.setFont(new Font("Helvetica", Font.PLAIN, this.fontSize));
this.fontSizeLabel.setText(Integer.toString(this.fontSize));
}
public static void main(String[] args) {
new FontSizer();
}
}
```
This Java program creates a window with a label for the letter "X" and a label for the font size value. It also has two buttons at the bottom to increase or decrease the font size. The font size of the "X" label and the font size value label are updated whenever the user clicks on the increase or decrease buttons.
To use this program, save the code in a file with a ".java" extension and compile it using a Java compiler. Then, run the program using the Java Virtual Machine (JVM).
阅读全文
相关推荐
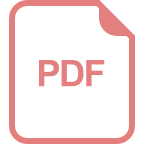
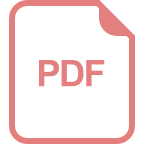
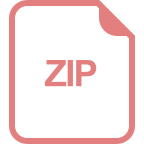
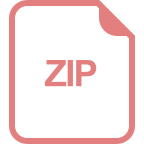
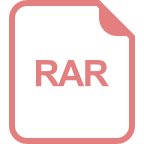
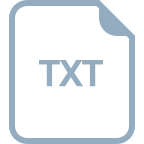
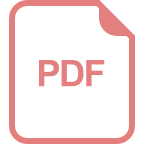
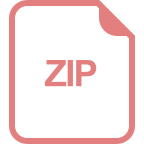
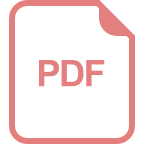
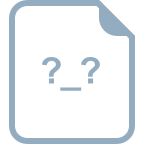
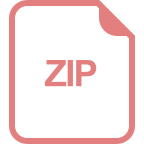
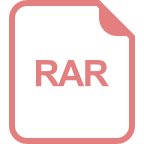
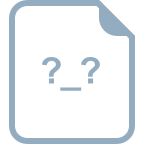
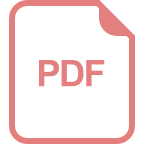