编写程序,从键盘输入5个学生的学生信息,包括学号、姓名、3门课程的成绩,计算3门课的平均成绩,将全部信息以二进制方式保存到文件中。
时间: 2023-09-09 15:04:21 浏览: 199
### 回答1:
以下是Python的实现:
```python
import struct
class Student:
def __init__(self, id, name, score1, score2, score3):
self.id = id
self.name = name
self.score1 = score1
self.score2 = score2
self.score3 = score3
def avg_score(self):
return (self.score1 + self.score2 + self.score3) / 3
def to_bytes(self):
id_bytes = struct.pack('i', self.id)
name_bytes = self.name.encode('utf-8')
name_len_bytes = struct.pack('i', len(name_bytes))
score1_bytes = struct.pack('f', self.score1)
score2_bytes = struct.pack('f', self.score2)
score3_bytes = struct.pack('f', self.score3)
return id_bytes + name_len_bytes + name_bytes + score1_bytes + score2_bytes + score3_bytes
students = []
for i in range(5):
id = int(input("学号: "))
name = input("姓名: ")
score1 = float(input("第一门课成绩: "))
score2 = float(input("第二门课成绩: "))
score3 = float(input("第三门课成绩: "))
students.append(Student(id, name, score1, score2, score3))
with open('students.bin', 'wb') as f:
for student in students:
f.write(student.to_bytes())
```
这里我们定义了一个`Student`类来存储学生信息,并且定义了一个`to_bytes`方法来将学生信息转换为二进制数据。然后我们循环输入5个学生的信息,并将它们存储在一个列表中。最后,我们将每个学生的二进制数据写入到文件`students.bin`中。
### 回答2:
编写该程序可以分为以下几个步骤:
1. 定义一个学生类,包括学号、姓名和3门课程的成绩,并在学生类中添加一个方法来计算3门课程的平均成绩。
```python
class Student:
def __init__(self, student_id, name, score1, score2, score3):
self.student_id = student_id
self.name = name
self.score1 = score1
self.score2 = score2
self.score3 = score3
def calc_average_score(self):
return (self.score1 + self.score2 + self.score3) / 3
```
2. 创建一个列表,用于保存输入的5个学生信息。
```python
students = []
```
3. 使用循环从键盘输入5个学生的学生信息,并添加到学生列表中。
```python
for i in range(5):
student_id = input("请输入学生学号:")
name = input("请输入学生姓名:")
score1 = float(input("请输入第1门课程成绩:"))
score2 = float(input("请输入第2门课程成绩:"))
score3 = float(input("请输入第3门课程成绩:"))
student = Student(student_id, name, score1, score2, score3)
students.append(student)
```
4. 创建一个二进制文件,并将学生列表以二进制方式保存到文件中。
```python
with open("student_data.bin", "wb") as file:
for student in students:
file.write(student.student_id.encode())
file.write(student.name.encode())
file.write(struct.pack('f', student.score1))
file.write(struct.pack('f', student.score2))
file.write(struct.pack('f', student.score3))
```
这样,程序就可以从键盘输入5个学生的学生信息,并将全部信息以二进制方式保存到名为`student_data.bin`的文件中。
### 回答3:
编写该程序需要使用到基本的输入输出函数和数据结构的知识。以下是一个使用Python语言实现的示例代码:
```python
import pickle
# 定义学生类
class Student:
def __init__(self, student_id, name, score1, score2, score3):
self.student_id = student_id
self.name = name
self.score1 = score1
self.score2 = score2
self.score3 = score3
def average_score(self):
return (self.score1 + self.score2 + self.score3) / 3
# 从键盘输入5个学生的信息
students = []
for i in range(5):
student_id = input("请输入第{}个学生的学号:".format(i+1))
name = input("请输入第{}个学生的姓名:".format(i+1))
score1 = int(input("请输入第{}个学生的第1门课的成绩:".format(i+1)))
score2 = int(input("请输入第{}个学生的第2门课的成绩:".format(i+1)))
score3 = int(input("请输入第{}个学生的第3门课的成绩:".format(i+1)))
student = Student(student_id, name, score1, score2, score3)
students.append(student)
# 计算每个学生的平均成绩
for student in students:
average_score = student.average_score()
print("学生{}的平均成绩为:{:.2f}".format(student.name, average_score))
# 将学生信息以二进制方式保存到文件中
with open('students.bin', 'wb') as file:
pickle.dump(students, file)
```
在程序中,我们首先定义了一个`Student`类来表示学生的基本信息和成绩,其中包括学号、姓名和3门课程的成绩。该类还包括一个方法`average_score`用于计算学生的平均成绩。
然后,我们使用一个循环从键盘输入了5个学生的信息,并创建了相应的`Student`对象,将它们存储在一个列表`students`中。
接着,我们遍历学生列表,分别计算每个学生的平均成绩,并将结果输出到屏幕上。
最后,我们使用`pickle`模块的`dump`函数将`students`列表以二进制方式保存到名为`students.bin`的文件中。需要注意的是,文件的扩展名`.bin`只是示例,您可以根据需要使用不同的扩展名。
希望以上回答能满足您的需求,如有不明之处,请随时追问。
阅读全文
相关推荐
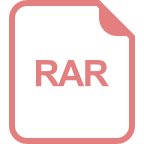













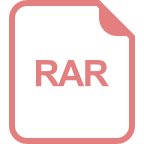
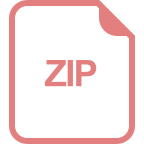