vue封装一个弹框组件 表格
时间: 2023-09-03 10:15:28 浏览: 115
好的,以下是一个简单的示例代码,供参考:
```vue
<template>
<div>
<button @click="showDialog">打开弹框</button>
<Dialog v-model="dialogVisible">
<h2 slot="title">标题</h2>
<div slot="content">
<Table :columns="columns" :data="tableData"></Table>
</div>
</Dialog>
</div>
</template>
<script>
import Dialog from './Dialog.vue'
import Table from './Table.vue'
export default {
components: { Dialog, Table },
data() {
return {
dialogVisible: false,
tableData: [
{ name: '张三', age: 20, job: 'Web 开发工程师' },
{ name: '李四', age: 25, job: '移动端开发工程师' },
{ name: '王五', age: 30, job: '前端架构师' },
],
columns: [
{ title: '姓名', key: 'name' },
{ title: '年龄', key: 'age' },
{ title: '职业', key: 'job' },
],
}
},
methods: {
showDialog() {
this.dialogVisible = true
},
},
}
</script>
```
其中,Dialog 和 Table 是两个子组件,代码如下:
```vue
<!-- Dialog.vue -->
<template>
<div class="dialog-mask" v-show="value">
<div class="dialog">
<div class="dialog-header">
<slot name="title">提示</slot>
<button class="dialog-close" @click="close">
<i class="el-icon-close"></i>
</button>
</div>
<div class="dialog-body">
<slot name="content"></slot>
</div>
</div>
</div>
</template>
<script>
export default {
props: {
value: {
type: Boolean,
default: false,
},
},
methods: {
close() {
this.$emit('input', false)
},
},
}
</script>
<style>
.dialog-mask {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5);
z-index: 999;
}
.dialog {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 500px;
height: 300px;
background-color: #fff;
border-radius: 4px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.2);
}
.dialog-header {
display: flex;
align-items: center;
justify-content: space-between;
padding: 10px;
font-size: 20px;
font-weight: bold;
border-bottom: 1px solid #eee;
}
.dialog-close {
border: none;
background-color: transparent;
font-size: 16px;
color: #999;
cursor: pointer;
outline: none;
}
.dialog-close:hover {
color: #333;
}
.dialog-body {
padding: 10px;
overflow: auto;
}
</style>
```
```vue
<!-- Table.vue -->
<template>
<table>
<thead>
<tr>
<th v-for="column in columns" :key="column.key">{{ column.title }}</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in data" :key="index">
<td v-for="column in columns" :key="column.key">{{ item[column.key] }}</td>
</tr>
</tbody>
</table>
</template>
<script>
export default {
props: {
columns: {
type: Array,
default: () => [],
},
data: {
type: Array,
default: () => [],
},
},
}
</script>
<style>
table {
width: 100%;
border-collapse: collapse;
}
th,
td {
padding: 10px;
border: 1px solid #eee;
}
</style>
```
以上代码仅供参考,具体实现还需要根据实际需求进行调整。
阅读全文
相关推荐
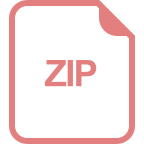
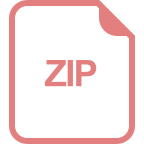
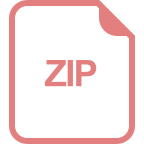
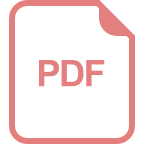
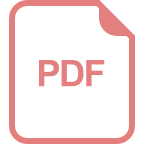
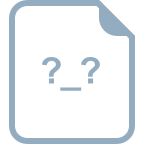
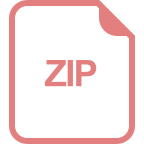
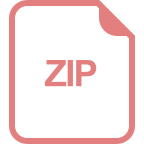
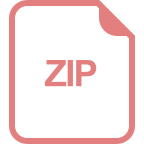
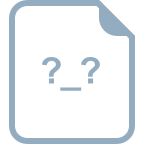
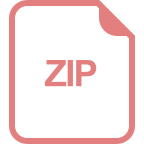
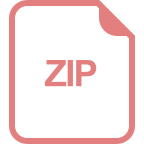
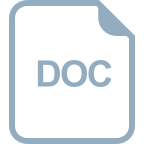
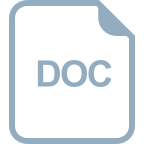
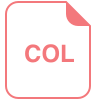

