mybatisplus如何用注解设置联合主键
时间: 2023-10-02 11:14:17 浏览: 6091
Mybatis Plus支持使用注解来设置联合主键,可以使用`@TableId`注解结合`value`和`type`属性来实现。具体操作步骤如下:
1. 在实体类中定义联合主键属性,可以使用`@TableId`注解指定其为主键,并设置`value`和`type`属性。
```java
@Data
@TableName("user_role")
public class UserRole {
@TableId(value = "user_id", type = IdType.INPUT)
private Long userId;
@TableId(value = "role_id", type = IdType.INPUT)
private Long roleId;
}
```
2. 在Mapper接口中继承`BaseMapper`接口,并指定实体类类型。
```java
@Mapper
public interface UserRoleMapper extends BaseMapper<UserRole> {
}
```
3. 在Mapper.xml文件中,使用`<insert>`、`<update>`和`<delete>`标签时,需要使用`<if>`标签来判断联合主键是否为空。同时,使用`<select>`标签查询时,需要使用`<where>`标签来拼接联合主键条件。
```xml
<insert id="insertUserRole" parameterType="com.example.UserRole">
insert into user_role (user_id, role_id)
values (#{userId}, #{roleId})
</insert>
<delete id="deleteUserRole" parameterType="com.example.UserRole">
delete from user_role
<where>
<if test="userId != null">and user_id = #{userId}</if>
<if test="roleId != null">and role_id = #{roleId}</if>
</where>
</delete>
<update id="updateUserRole" parameterType="com.example.UserRole">
update user_role
<set>
<if test="roleId != null">role_id = #{roleId},</if>
</set>
<where>
<if test="userId != null">and user_id = #{userId}</if>
</where>
</update>
<select id="getUserRoleById" parameterType="com.example.UserRole" resultType="com.example.UserRole">
select *
from user_role
<where>
<if test="userId != null">and user_id = #{userId}</if>
<if test="roleId != null">and role_id = #{roleId}</if>
</where>
</select>
```
通过以上步骤,即可使用注解设置联合主键,并通过Mybatis Plus进行CRUD操作。
阅读全文
相关推荐
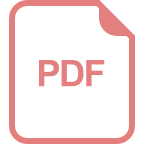
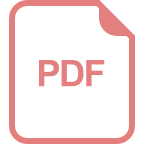








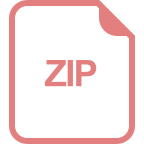
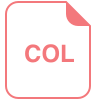


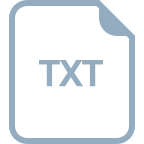
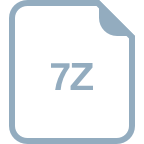