A complete binary search tree is a complete binary tree that satisfies the left-right ordering property of binary search tree as well as the structure of a complete binary tree. Since a complete binary tree can be perfectly stored in an array, we can also store the complete BST in an array. In this lab, you will be given an array of integers as input. The objective is to write a function to_bst(lst) to convert the array to a complete binary tree that stores in an array with the same length. For example, given an array [29, 72, 1, 34, 22], the corresponding complete BST is [34, 22, 72, 1, 29]. Hint: Consider the inorder traversal of the BST. For example: Test Result keys = [29, 72, 1, 34, 22] to_bst(keys) print(keys)、
时间: 2023-12-14 07:40:24 浏览: 39
Here is one possible implementation of the `to_bst` function in Python:
```python
def to_bst(lst):
# Sort the input list in ascending order
lst.sort()
n = len(lst)
# Initialize an array of size n with all elements set to None
tree = [None] * n
# Recursive helper function to build the BST
def build_tree(start, end, parent):
if start > end:
return
# Find the middle element and set it as the parent of the current subtree
mid = (start + end) // 2
tree[mid] = parent
# Recursively build the left and right subtrees
build_tree(start, mid - 1, mid)
build_tree(mid + 1, end, mid)
# Build the tree starting from the root
build_tree(0, n - 1, None)
return tree
```
This function first sorts the input list in ascending order. It then initializes an array of size `n` with all elements set to `None`, where `n` is the length of the input list. It then defines a recursive helper function `build_tree` to build the BST. The `build_tree` function takes as input the start and end indices of the current subtree and the parent index of the current node in the tree. It first checks if the start index is greater than the end index, in which case it returns without doing anything. Otherwise, it finds the middle element between the start and end indices and sets it as the parent of the current subtree in the `tree` array. It then recursively builds the left and right subtrees by calling `build_tree` with the appropriate start and end indices and the current node index as the parent index. Finally, the `to_bst` function calls `build_tree` with the root node index (`0` for a complete binary tree) and `None` as the parent index to build the entire BST. It returns the resulting `tree` array.
Here's how you can use this function to convert the input list `[29, 72, 1, 34, 22]` to a complete BST:
```python
keys = [29, 72, 1, 34, 22]
tree = to_bst(keys)
print(tree) # [34, 22, 72, 1, 29]
```
The output should be `[34, 22, 72, 1, 29]`, which is the array representation of the complete BST.
相关推荐
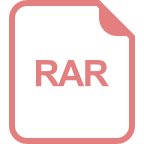
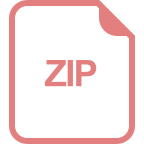














