springcloudgateway限流熔断
时间: 2023-09-21 21:04:37 浏览: 142
Spring Cloud Gateway 提供了限流和熔断的功能来保护后端服务免受过载的影响。下面是一些常见的方法来实现限流和熔断:
1. 限流:可以使用 Spring Cloud Gateway 的内置限流过滤器来实现限流功能。可以配置每秒钟允许通过的请求数量,并根据需要进行调整。使用限流可以保护后端服务免受请求过多的影响,确保服务的稳定性。
2. 熔断:可以使用 Spring Cloud Gateway 的熔断过滤器来实现熔断功能。当后端服务出现故障或响应时间过长时,可以将请求快速失败,避免对后端服务的进一步压力。可以配置熔断的阈值和时间窗口,以及熔断后的重试策略。
要实现限流和熔断功能,可以在 Spring Cloud Gateway 的配置文件中配置相应的过滤器,并设置相关的参数。同时,可以使用 Spring Cloud Circuit Breaker 来提供更强大的熔断功能,如基于失败率的熔断等。
需要注意的是,限流和熔断只是保护后端服务免受过载的影响,并不能解决服务故障或性能问题的根本原因。因此,在实施限流和熔断之前,需要对后端服务进行充分的性能测试和故障排查,以保证服务的质量和可靠性。
相关问题
springcloud gateway 熔断 降级 限流
Spring Cloud Gateway 提供了熔断、降级和限流等功能,可以让应用在高并发、异常流量等情况下保持稳定性。
1. 熔断
熔断是指在服务异常或故障的情况下,保护系统不受影响的一种机制。当服务出现异常或故障时,会触发熔断器,阻止请求到达服务,从而保护服务和系统的稳定性。
Spring Cloud Gateway 提供了 Hystrix 熔断器的支持,可以通过配置熔断器来实现熔断功能。
2. 降级
降级是指在系统资源不足或异常流量过大的情况下,通过限制部分功能或服务的使用,保证系统的核心功能能够正常运行。
Spring Cloud Gateway 通过配置路由规则,可以实现针对不同场景的降级策略。
3. 限流
限流是指在高并发场景下,通过限制请求的速率或数量,保护系统不受过载的一种机制。
Spring Cloud Gateway 提供了基于令牌桶算法的限流功能,可以通过配置限流规则来实现限流。
详细说明spring cloud hystrix的限流,熔断,降级并给出代码和配置示例。详细说明spring cloud gateway熔断,限流和降级并给出代码和示例
一、Spring Cloud Hystrix
1. 什么是 Hystrix
Hystrix 是一个延迟和容错库,用于隔离依赖服务的访问点,以防止这些依赖服务的故障导致雪崩效应。它提供了熔断、限流和降级等机制,保障了对依赖服务的访问。
2. Hystrix的核心概念
熔断:在一段时间内,如果服务的错误比例超过了设定的阈值,那么这个服务就会被熔断,示例代码:
```
@HystrixCommand(fallbackMethod = "fallbackMethod")
public String method() {
int i = new Random().nextInt(10);
if (i % 2 == 0) {
throw new RuntimeException("error");
}
return "success";
}
public String fallbackMethod(){
return "fallback";
}
```
限流:在一段时间内,如果服务的请求数量超过了设定的阈值,那么这个服务就会被限流,示例代码:
```
@HystrixCommand(fallbackMethod = "fallbackMethod", commandProperties = {
@HystrixProperty(name = "execution.isolation.thread.timeoutInMilliseconds", value = "500"),
@HystrixProperty(name = "circuitBreaker.requestVolumeThreshold", value = "10"),
@HystrixProperty(name = "circuitBreaker.errorThresholdPercentage", value = "50"),
@HystrixProperty(name = "circuitBreaker.sleepWindowInMilliseconds", value = "30000")})
public String method() {
return "success";
}
public String fallbackMethod(){
return "fallback";
}
```
降级:在一段时间内,如果依赖服务不可用,那么就会调用预先备选的服务逻辑或返回预先设定的响应,示例代码:
```
@HystrixCommand(fallbackMethod = "fallbackMethod")
public String method() {
return restTemplate.getForObject("http://service-provider/method", String.class);
}
public String fallbackMethod(){
return "fallback";
}
```
3. Hystrix的集成
添加依赖
```
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix</artifactId>
</dependency>
```
开启熔断
```
@SpringBootApplication
@EnableCircuitBreaker
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
添加注解
```
@Service
public class Service {
@HystrixCommand(fallbackMethod = "fallbackMethod")
public String method() {
return "success";
}
public String fallbackMethod() {
return "fallback";
}
}
```
4. 测试
启动服务后,访问 http://localhost:8080/method,可以看到服务正常返回"success"。将calledOnce设置为false并再次访问该URL,可以看到服务返回"fallback"。
二、Spring Cloud Gateway
1. 什么是 Gateway
Gateway 是 Spring Cloud 提供的一种 API 网关服务,基于 Spring 5.0、Spring Boot 2.0 和 Project Reactor 等技术开发。
2. Gateway 的三种机制
熔断:默认使用 Hystrix 进行熔断,示例代码:
```
spring.cloud.gateway.routes.id=route1
spring.cloud.gateway.routes.uri=http://httpbin.org:80
spring.cloud.gateway.routes.predicate[0]=Host=**.somehost.org
spring.cloud.gateway.routes.predicate[1]=Path=/get
spring.cloud.gateway.routes.filters[0]=Hystrix=hystrixCommandName
```
限流:使用 RequestRateLimiter Gateway Filter 进行限流,示例代码:
```
spring.cloud.gateway.routes[0].id=count_route
spring.cloud.gateway.routes[0].uri=http://httpbin.org:80
spring.cloud.gateway.routes[0].predicates[0]=Path=/get
spring.cloud.gateway.routes[0].filters[0]=RequestRateLimiter=my-limiter-key,2,10,PT1S
```
降级:可以使用 Hystrix 进行降级,示例代码:
```
spring.cloud.gateway.routes[0].id=test_route
spring.cloud.gateway.routes[0].uri=http://localhost:9090
spring.cloud.gateway.routes[0].predicates[0]=Path=/test
spring.cloud.gateway.routes[0].filters[0]=Hystrix=mycommand
```
3. Gateway 的集成
添加依赖
```
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway</artifactId>
</dependency>
```
4. 测试
启动服务后,访问 http://localhost:8080/get,可以看到服务正常返回。将请求频率配置为 0.1s,再次访问该 URL,可以看到服务返回 429 Too Many Requests。
参考资料:
1. Spring Cloud Hystrix 官方文档:https://cloud.spring.io/spring-cloud-static/spring-cloud-netflix/2.2.1.RELEASE/reference/html/#spring-cloud-hystrix
2. Spring Cloud Gateway 官方文档:https://cloud.spring.io/spring-cloud-static/spring-cloud-gateway/2.2.1.RELEASE/reference/html/
阅读全文
相关推荐
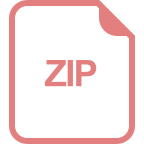
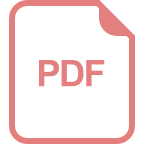
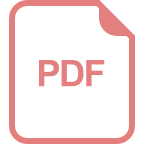
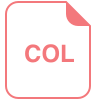
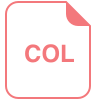
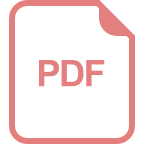
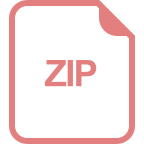
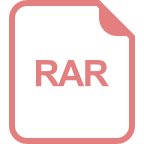
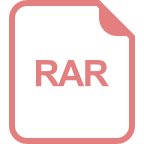
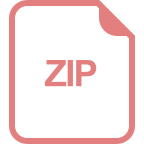
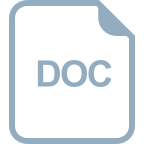
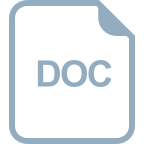
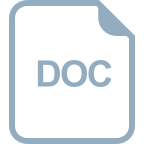
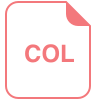
