java查看已上传的文件夹_SpringMVC文件上传及查看的示例代码
时间: 2024-03-07 17:49:01 浏览: 57
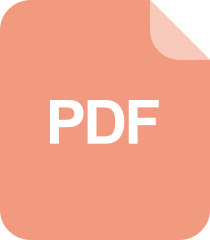
Java/Web调用Hadoop进行MapReduce示例代码
以下是SpringMVC文件上传及查看的示例代码:
1. 文件上传:
```java
@Controller
@RequestMapping("/file")
public class FileController {
@RequestMapping(value = "/upload", method = RequestMethod.POST)
@ResponseBody
public String upload(@RequestParam("file") MultipartFile file) {
try {
// 保存文件
String fileName = file.getOriginalFilename();
String filePath = "/path/to/save/" + fileName;
File dest = new File(filePath);
file.transferTo(dest);
return "success";
} catch (Exception e) {
e.printStackTrace();
return "error";
}
}
}
```
2. 文件查看:
```java
@Controller
@RequestMapping("/file")
public class FileController {
@RequestMapping("/list")
public String list(Model model) {
// 获取文件列表
String fileDirPath = "/path/to/list/";
File fileDir = new File(fileDirPath);
File[] fileList = fileDir.listFiles();
List<String> fileNameList = new ArrayList<>();
for (File file : fileList) {
fileNameList.add(file.getName());
}
model.addAttribute("fileNameList", fileNameList);
return "file/list";
}
@RequestMapping("/download/{fileName}")
public void download(@PathVariable("fileName") String fileName, HttpServletResponse response) {
// 下载文件
String filePath = "/path/to/download/" + fileName;
try {
FileInputStream fis = new FileInputStream(filePath);
response.setContentType("application/force-download");
response.addHeader("Content-Disposition", "attachment;fileName=" + fileName);
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
response.getOutputStream().write(buffer, 0, len);
}
fis.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
其中,list方法用于获取文件列表,download方法用于下载文件。注意,download方法中设置了响应头,告诉浏览器下载的文件名字,并将文件内容写入响应体。
阅读全文
相关推荐
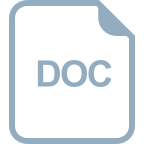
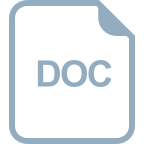
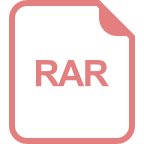
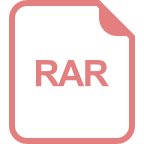
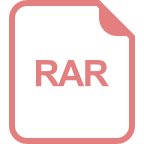
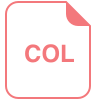
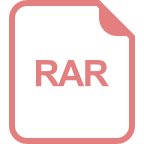
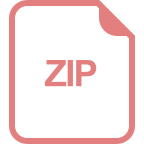
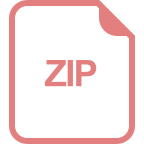
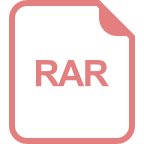
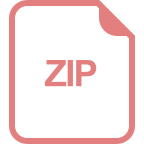
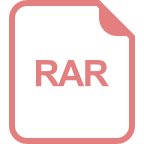
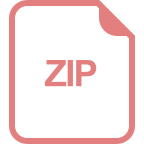
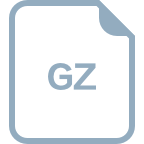
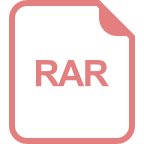
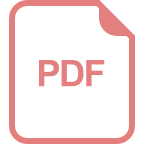

