Java MultipartFile实现文件上传
时间: 2023-10-13 17:29:06 浏览: 127
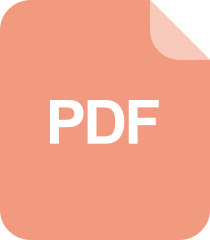
利用MultipartFile实现文件上传功能

Java 中的 MultipartFile 接口用于对文件进行操作,实现文件上传。
以下是一个简单的文件上传示例,其中包含一个 HTML 表单和一个 SpringMVC 的控制器:
HTML 表单:
```html
<!DOCTYPE html>
<html>
<head>
<title>文件上传</title>
</head>
<body>
<h2>文件上传</h2>
<form action="http://localhost:8080/upload" method="POST" enctype="multipart/form-data">
<input type="file" name="file" />
<br /><br />
<input type="submit" value="上传" />
</form>
</body>
</html>
```
SpringMVC 控制器:
```java
@Controller
public class FileUploadController {
@PostMapping("/upload")
public ResponseEntity<String> handleFileUpload(@RequestParam("file") MultipartFile file) {
String fileName = StringUtils.cleanPath(file.getOriginalFilename());
try {
if (file.isEmpty()) {
throw new FileNotFoundException("请上传文件!");
}
if (fileName.contains("..")) {
throw new FileNotFoundException("文件名无效!");
}
// 将文件保存到本地磁盘
Path path = Paths.get("/path/to/save/" + fileName);
Files.copy(file.getInputStream(), path, StandardCopyOption.REPLACE_EXISTING);
} catch (IOException e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body(e.getMessage());
}
return ResponseEntity.ok().body("文件上传成功!");
}
}
```
在控制器中,使用 `@RequestParam` 注解获取 `file` 参数,并使用 `MultipartFile` 对象进行操作。在 `handleFileUpload` 方法中,首先检查文件是否存在以及文件名是否有效。然后,使用 `Files.copy` 方法将文件保存到本地磁盘。
注意:本示例中的保存路径为 `/path/to/save/`,需要替换为实际的保存路径。
阅读全文
相关推荐














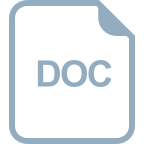
