采用c语言实现一“图书管理系统〞,为用户提供管理和查询服务。 图书信息包括8个数据项:书名,ISBN、作者、出版社、出版时间,入库时间, 库存量(图书馆购买此书的数量),借阅数(被借阅的数量)等信息。完成以下 操作: (1)录入:图书信息(至少10条记录): (2)删除:删除某一本图书的信息; (3)修改:修改某一本图书的信息: (4)查询:根据书名或图书ISBV等查询图书信息(查询方法不限)。 (5)借书:借出一本书,该书的借阅数增加一本。 (6)还书:还回一本书,该书的借阅数减少一本。 (7)统计:统计所有借出的图书数目,即所有图书借阅数之和。 (8)输出:输出所查询图书的信息,要求格式清晰,方便查看。
时间: 2023-12-03 19:42:27 浏览: 31
这是一个比较完整的项目,需要设计数据结构和相关操作函数。下面是一个简单的实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define MAX_BOOKS 100 // 最大书籍数量
#define MAX_NAME 50 // 最大书名长度
#define MAX_ISBN 20 // 最大ISBN长度
#define MAX_AUTHOR 30 // 最大作者名长度
#define MAX_PUBLISHER 30 // 最大出版社名长度
typedef struct Book {
char name[MAX_NAME];
char isbn[MAX_ISBN];
char author[MAX_AUTHOR];
char publisher[MAX_PUBLISHER];
time_t publish_date;
time_t enter_date;
int stock;
int borrow_count;
} Book;
Book books[MAX_BOOKS];
int book_count = 0;
void add_book() {
if (book_count < MAX_BOOKS) {
Book book;
printf("请输入书名:");
scanf("%s", book.name);
printf("请输入ISBN:");
scanf("%s", book.isbn);
printf("请输入作者名:");
scanf("%s", book.author);
printf("请输入出版社名:");
scanf("%s", book.publisher);
printf("请输入出版时间(格式为YYYY-MM-DD):");
char date_str[11];
scanf("%s", date_str);
struct tm publish_date_tm;
strptime(date_str, "%Y-%m-%d", &publish_date_tm);
book.publish_date = mktime(&publish_date_tm);
book.enter_date = time(NULL);
printf("请输入库存量:");
scanf("%d", &book.stock);
book.borrow_count = 0;
books[book_count++] = book;
printf("添加成功!\n");
} else {
printf("无法添加,书籍数量已达上限。\n");
}
}
void delete_book() {
char isbn[MAX_ISBN];
printf("请输入要删除的书籍的ISBN号:");
scanf("%s", isbn);
int index = -1;
for (int i = 0; i < book_count; i++) {
if (strcmp(isbn, books[i].isbn) == 0) {
index = i;
break;
}
}
if (index >= 0) {
for (int i = index; i < book_count - 1; i++) {
books[i] = books[i + 1];
}
book_count--;
printf("删除成功!\n");
} else {
printf("找不到该书籍。\n");
}
}
void modify_book() {
char isbn[MAX_ISBN];
printf("请输入要修改的书籍的ISBN号:");
scanf("%s", isbn);
int index = -1;
for (int i = 0; i < book_count; i++) {
if (strcmp(isbn, books[i].isbn) == 0) {
index = i;
break;
}
}
if (index >= 0) {
printf("请输入新的书名(留空表示不修改):");
char new_name[MAX_NAME];
scanf("%s", new_name);
if (strlen(new_name) > 0) {
strcpy(books[index].name, new_name);
}
printf("请输入新的作者名(留空表示不修改):");
char new_author[MAX_AUTHOR];
scanf("%s", new_author);
if (strlen(new_author) > 0) {
strcpy(books[index].author, new_author);
}
printf("请输入新的出版社名(留空表示不修改):");
char new_publisher[MAX_PUBLISHER];
scanf("%s", new_publisher);
if (strlen(new_publisher) > 0) {
strcpy(books[index].publisher, new_publisher);
}
printf("请输入新的出版时间(留空表示不修改,格式为YYYY-MM-DD):");
char date_str[11];
scanf("%s", date_str);
if (strlen(date_str) > 0) {
struct tm publish_date_tm;
strptime(date_str, "%Y-%m-%d", &publish_date_tm);
books[index].publish_date = mktime(&publish_date_tm);
}
printf("请输入新的库存量(留空表示不修改):");
char stock_str[11];
scanf("%s", stock_str);
if (strlen(stock_str) > 0) {
books[index].stock = atoi(stock_str);
}
printf("修改成功!\n");
} else {
printf("找不到该书籍。\n");
}
}
void search_book() {
char keyword[MAX_NAME];
printf("请输入要查询的关键字(书名或ISBN号):");
scanf("%s", keyword);
int found_count = 0;
for (int i = 0; i < book_count; i++) {
if (strstr(books[i].name, keyword) != NULL ||
strstr(books[i].isbn, keyword) != NULL) {
printf("书名:%s\nISBN:%s\n作者:%s\n出版社:%s\n出版时间:%s\n库存量:%d\n借阅数:%d\n\n",
books[i].name, books[i].isbn, books[i].author, books[i].publisher,
ctime(&books[i].publish_date), books[i].stock, books[i].borrow_count);
found_count++;
}
}
if (found_count == 0) {
printf("找不到符合条件的书籍。\n");
}
}
void borrow_book() {
char isbn[MAX_ISBN];
printf("请输入要借阅的书籍的ISBN号:");
scanf("%s", isbn);
int index = -1;
for (int i = 0; i < book_count; i++) {
if (strcmp(isbn, books[i].isbn) == 0) {
index = i;
break;
}
}
if (index >= 0) {
if (books[index].stock > 0) {
books[index].stock--;
books[index].borrow_count++;
printf("借阅成功!\n");
} else {
printf("该书籍库存不足,无法借阅。\n");
}
} else {
printf("找不到该书籍。\n");
}
}
void return_book() {
char isbn[MAX_ISBN];
printf("请输入要归还的书籍的ISBN号:");
scanf("%s", isbn);
int index = -1;
for (int i = 0; i < book_count; i++) {
if (strcmp(isbn, books[i].isbn) == 0) {
index = i;
break;
}
}
if (index >= 0) {
if (books[index].borrow_count > 0) {
books[index].stock++;
books[index].borrow_count--;
printf("归还成功!\n");
} else {
printf("该书籍没有被借阅,无法归还。\n");
}
} else {
printf("找不到该书籍。\n");
}
}
void count_borrowed_books() {
int total = 0;
for (int i = 0; i < book_count; i++) {
total += books[i].borrow_count;
}
printf("共借出%d本书籍。\n", total);
}
int main() {
int choice;
while (1) {
printf("请选择操作:\n");
printf("1. 录入书籍\n");
printf("2. 删除书籍\n");
printf("3. 修改书籍\n");
printf("4. 查询书籍\n");
printf("5. 借阅书籍\n");
printf("6. 归还书籍\n");
printf("7. 统计借阅数\n");
printf("0. 退出程序\n");
printf("请输入操作编号:");
scanf("%d", &choice);
switch (choice) {
case 1:
add_book();
break;
case 2:
delete_book();
break;
case 3:
modify_book();
break;
case 4:
search_book();
break;
case 5:
borrow_book();
break;
case 6:
return_book();
break;
case 7:
count_borrowed_books();
break;
case 0:
printf("谢谢使用!\n");
return 0;
default:
printf("无效操作,请重新输入。\n");
}
}
}
```
这个程序可以在控制台中运行,根据提示进行各种操作。当然,这只是一个简单的示例,实际的图书管理系统需要更完善的功能和更健壮的代码。
相关推荐
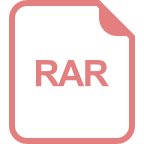
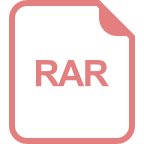
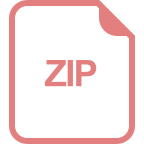
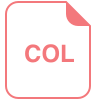
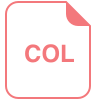
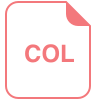
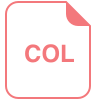
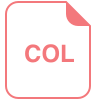









