使用java设计如下界面,在界面上方有一个标签“I’m a able”,中间有一个随机颜色的圆形,下方有两个按钮,点击点击“change lable”后标签改变为“ouch!”。点击“change circle”后圆改变颜色。
时间: 2024-03-11 17:46:51 浏览: 55
好的,以下是Java Swing实现的代码示例:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class MyFrame extends JFrame implements ActionListener {
private JLabel label;
private JButton labelButton;
private JButton circleButton;
private JPanel panel;
private Color circleColor;
public MyFrame() {
// 设置窗口标题
setTitle("My Frame");
// 创建标签
label = new JLabel("I'm able", JLabel.CENTER);
label.setFont(new Font("宋体", Font.PLAIN, 24));
label.setForeground(Color.BLACK);
// 创建按钮
labelButton = new JButton("Change Label");
labelButton.addActionListener(this);
circleButton = new JButton("Change Circle");
circleButton.addActionListener(this);
// 创建面板并添加组件
panel = new JPanel(new BorderLayout());
panel.add(label, BorderLayout.CENTER);
JPanel buttonPanel = new JPanel(new GridLayout(1, 2));
buttonPanel.add(labelButton);
buttonPanel.add(circleButton);
panel.add(buttonPanel, BorderLayout.SOUTH);
// 创建随机颜色的圆形
circleColor = getRandomColor();
CirclePanel circlePanel = new CirclePanel(circleColor);
panel.add(circlePanel, BorderLayout.NORTH);
// 添加面板到窗口
add(panel);
// 设置窗口大小并居中
setSize(400, 400);
setLocationRelativeTo(null);
}
// 按钮点击事件
public void actionPerformed(ActionEvent e) {
if (e.getSource() == labelButton) {
label.setText("Ouch!");
} else if (e.getSource() == circleButton) {
circleColor = getRandomColor();
panel.remove(0);
CirclePanel newCirclePanel = new CirclePanel(circleColor);
panel.add(newCirclePanel, BorderLayout.NORTH, 0);
panel.revalidate();
panel.repaint();
}
}
// 获取随机颜色
private Color getRandomColor() {
int r = (int) (Math.random() * 256);
int g = (int) (Math.random() * 256);
int b = (int) (Math.random() * 256);
return new Color(r, g, b);
}
// 圆形面板
private class CirclePanel extends JPanel {
private Color color;
public CirclePanel(Color color) {
this.color = color;
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(color);
g.fillOval(50, 50, 100, 100);
}
}
public static void main(String[] args) {
MyFrame frame = new MyFrame();
frame.setVisible(true);
}
}
```
运行该程序即可看到界面。点击“Change Label”按钮可以改变标签内容,点击“Change Circle”按钮可以改变圆形颜色。
阅读全文
相关推荐
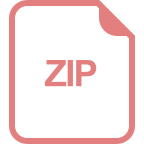
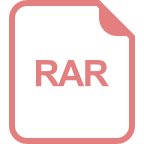
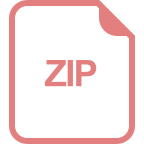
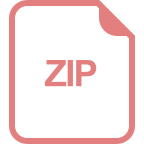
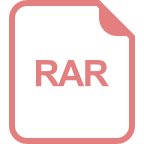
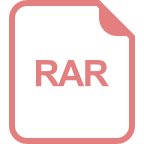
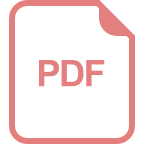
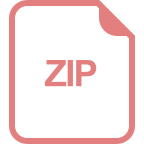
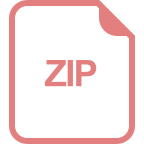
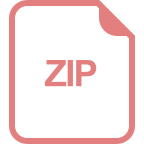
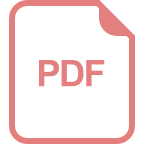
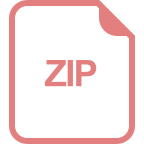
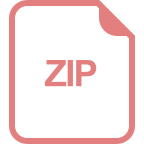
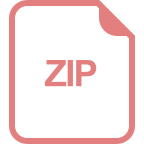
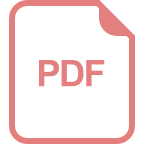
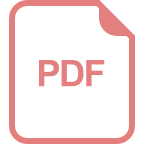
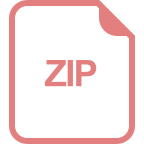