多层感知机的几种调参方法怎么代码实现
时间: 2023-08-18 10:10:41 浏览: 52
多层感知机(MLP)的调参方法有很多种,下面介绍一些常用的方法和对应的代码实现。
1. 网格搜索(Grid Search)
网格搜索通过枚举所有可能的超参数组合来寻找最优的超参数,代码如下:
```python
from sklearn.model_selection import GridSearchCV
from sklearn.neural_network import MLPClassifier
# 定义超参数范围
param_grid = {
'hidden_layer_sizes': [(50,), (100,), (150,), (200,)],
'activation': ['relu', 'logistic', 'tanh'],
'solver': ['sgd', 'adam'],
'learning_rate': ['constant', 'adaptive']
}
# 定义MLP模型
mlp = MLPClassifier()
# 定义GridSearchCV
grid_search = GridSearchCV(mlp, param_grid, cv=5)
# 训练模型并搜索最优超参数
grid_search.fit(X_train, y_train)
# 输出最优超参数和模型得分
print("Best parameters: ", grid_search.best_params_)
print("Best score: ", grid_search.best_score_)
```
在上面的代码中,我们使用了sklearn库中的GridSearchCV类来进行网格搜索。首先,我们定义了超参数的范围,包括隐藏层大小、激活函数、优化器和学习率。然后,我们通过MLPClassifier定义了一个多层感知机模型。接着,我们使用GridSearchCV来搜索最优超参数,并在训练集上进行5折交叉验证。最后,我们输出最优超参数和模型在交叉验证集上的得分。
2. 随机搜索(Random Search)
随机搜索通过随机采样超参数来寻找最优的超参数,代码如下:
```python
from sklearn.model_selection import RandomizedSearchCV
from sklearn.neural_network import MLPClassifier
from scipy.stats import randint as sp_randint
# 定义超参数范围
param_dist = {
'hidden_layer_sizes': sp_randint(50, 200),
'activation': ['relu', 'logistic', 'tanh'],
'solver': ['sgd', 'adam'],
'learning_rate': ['constant', 'adaptive']
}
# 定义MLP模型
mlp = MLPClassifier()
# 定义RandomizedSearchCV
random_search = RandomizedSearchCV(mlp, param_distributions=param_dist, n_iter=20, cv=5, random_state=42)
# 训练模型并搜索最优超参数
random_search.fit(X_train, y_train)
# 输出最优超参数和模型得分
print("Best parameters: ", random_search.best_params_)
print("Best score: ", random_search.best_score_)
```
在上面的代码中,我们使用了sklearn库中的RandomizedSearchCV类来进行随机搜索。和网格搜索类似,我们首先定义了超参数的范围,但是这里使用了scipy库中的randint函数来定义隐藏层大小的范围。接着,我们定义了MLP模型,并使用RandomizedSearchCV来进行随机搜索,设置了20次采样,并在训练集上进行5折交叉验证。最后,我们输出最优超参数和模型在交叉验证集上的得分。
3. 梯度下降法(Gradient Descent)
梯度下降法通过对损失函数进行求导,调整超参数来最小化损失函数,代码如下:
```python
from sklearn.neural_network import MLPClassifier
# 定义MLP模型
mlp = MLPClassifier(hidden_layer_sizes=(100,), activation='relu', solver='adam', learning_rate='constant', max_iter=1000)
# 训练模型并输出模型得分
mlp.fit(X_train, y_train)
print("Training set score: %f" % mlp.score(X_train, y_train))
print("Test set score: %f" % mlp.score(X_test, y_test))
# 调整超参数并重新训练模型
mlp.set_params(hidden_layer_sizes=(200,), max_iter=2000)
mlp.fit(X_train, y_train)
print("Training set score: %f" % mlp.score(X_train, y_train))
print("Test set score: %f" % mlp.score(X_test, y_test))
```
在上面的代码中,我们使用了sklearn库中的MLPClassifier类来定义MLP模型,并设置了一些超参数,包括1个隐藏层、ReLU激活函数、Adam优化器和常数学习率。接着,我们训练模型并输出训练集和测试集上的得分。然后,我们通过set_params函数调整了隐藏层大小和迭代次数,并重新训练模型。最后,我们输出了调整后的训练集和测试集得分。
需要注意的是,梯度下降法调参需要对超参数进行逐一调整,并观察模型在训练集和测试集上的表现,以确定最优的超参数组合。
相关推荐
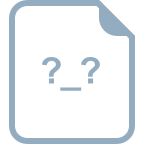
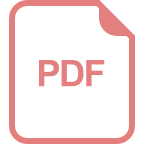













