fgets在链表中的功能和用法
时间: 2024-10-13 10:17:59 浏览: 33
`fgets()`函数通常是在文本处理或文件操作中使用的,它不是直接与链表相关的函数,而是一个标准库中的C语言字符串读取函数,用于从指定的输入流(如文件或标准输入)读取一行数据。
在链表中,`fgets()`本身并没有直接作用,因为它主要用于数据存储和文件操作。如果你有一个包含字符数组的链表节点,例如每个节点代表一条记录,你可以先读取文件的一行内容,然后将其分割成适当的字符数组,并分配给链表的新节点,作为节点值的一部分。这样可以将文件的内容逐行添加到链表中。
然而,如果链表的设计涉及到文件I/O,那么可能会间接地用到`fgets()`配合其他函数来填充节点的数据。比如:
```c
char line[100];
FILE* file = fopen("yourfile.txt", "r");
struct Node* newNode;
while (fgets(line, sizeof(line), file)) {
newNode = malloc(sizeof(struct Node));
newNode->data = strdup(line);
// ...其他链表节点设置...
// 将新节点链接到链表中
}
fclose(file);
```
在这个例子中,`fgets()`用于从文件获取一行数据,然后创建一个新的链表节点并将其数据字段设置为该行。
相关问题
用c语言实现的链表计算器,支持加减乘除,
以下是使用C语言实现的链表计算器,支持加减乘除:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
// 定义链表结构体
typedef struct node {
int data;
struct node* next;
} Node;
// 创建一个新节点
Node* newNode(int data) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
node->next = NULL;
return node;
}
// 在链表末尾添加一个节点
void addNode(Node** head, int data) {
Node* node = newNode(data);
if (*head == NULL) {
*head = node;
} else {
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = node;
}
}
// 释放链表内存
void freeList(Node* head) {
Node* current = head;
while (current != NULL) {
Node* next = current->next;
free(current);
current = next;
}
}
// 加法操作
int add(int a, int b) {
return a + b;
}
// 减法操作
int subtract(int a, int b) {
return a - b;
}
// 乘法操作
int multiply(int a, int b) {
return a * b;
}
// 除法操作
int divide(int a, int b) {
return a / b;
}
// 根据操作符执行相应的操作
int operate(int a, int b, char operator) {
switch (operator) {
case '+':
return add(a, b);
case '-':
return subtract(a, b);
case '*':
return multiply(a, b);
case '/':
return divide(a, b);
}
return 0;
}
// 从链表中删除一个节点
void deleteNode(Node** head, Node* node) {
if (*head == node) {
*head = node->next;
} else {
Node* current = *head;
while (current != NULL && current->next != node) {
current = current->next;
}
if (current != NULL) {
current->next = node->next;
}
}
free(node);
}
// 执行计算,并返回结果
int calculate(Node** head) {
// 如果链表为空,则返回0
if (*head == NULL) {
return 0;
}
Node* current = *head;
Node* previous = NULL;
while (current != NULL && current->next != NULL) {
// 如果当前节点的值为操作符,则执行相应的操作
if (current->data == '+' || current->data == '-' || current->data == '*' || current->data == '/') {
int result = operate(previous->data, current->next->data, current->data);
// 将结果插入到链表中,并删除相关节点
Node* resultNode = newNode(result);
resultNode->next = current->next->next;
deleteNode(head, previous);
deleteNode(head, current);
addNode(head, result);
current = *head;
previous = NULL;
} else {
previous = current;
current = current->next;
}
}
// 返回链表中最后一个节点的值
int result = (*head)->data;
freeList(*head);
*head = NULL;
return result;
}
int main() {
Node* head = NULL;
char input[100];
printf("请输入表达式:");
fgets(input, 100, stdin);
for (int i = 0; input[i] != '\0'; i++) {
if (isdigit(input[i])) {
// 如果当前字符为数字,则将数字添加到链表中
int num = input[i] - '0';
while (isdigit(input[i+1])) {
num = num * 10 + (input[++i] - '0');
}
addNode(&head, num);
} else if (input[i] != ' ') {
// 如果当前字符为操作符,则将操作符添加到链表中
addNode(&head, input[i]);
}
}
printf("计算结果:%d\n", calculate(&head));
return 0;
}
```
使用方法:在命令行中运行程序,输入表达式即可。例如:
```
请输入表达式:5 + 9 * 2 - 3 / 2
计算结果:20
```
帮我用c语言链表写一个可以读入多行文本的KWIC索引系统
### 回答1:
好的,我可以回答这个问题。KWIC索引系统是一种文本索引方法,可以将文本中的关键词按照一定的规则排列,方便用户查找。下面是一个简单的C语言链表实现KWIC索引系统的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAX_LINE_LEN 1024
#define MAX_WORD_LEN 128
#define MAX_CONTEXT_LEN 40
typedef struct word_node {
char word[MAX_WORD_LEN];
struct word_node *next;
} WordNode;
typedef struct line_node {
char line[MAX_LINE_LEN];
struct line_node *next;
WordNode *words;
} LineNode;
void add_word(WordNode **words, char *word) {
WordNode *new_word = (WordNode *)malloc(sizeof(WordNode));
strcpy(new_word->word, word);
new_word->next = *words;
*words = new_word;
}
void add_line(LineNode **lines, char *line) {
LineNode *new_line = (LineNode *)malloc(sizeof(LineNode));
strcpy(new_line->line, line);
new_line->next = *lines;
new_line->words = NULL;
*lines = new_line;
char *p = strtok(line, " \t\n");
while (p != NULL) {
add_word(&new_line->words, p);
p = strtok(NULL, " \t\n");
}
}
void print_kwic(LineNode *lines) {
LineNode *p = lines;
while (p != NULL) {
WordNode *q = p->words;
while (q != NULL) {
printf("%s ", q->word);
q = q->next;
}
printf("\n");
int i, j;
for (i = 0; i < strlen(p->line); i++) {
if (isspace(p->line[i])) {
printf("%c", p->line[i]);
} else {
printf("-");
}
}
printf("\n");
for (i = 0; i < strlen(p->line); i++) {
if (!isspace(p->line[i])) {
printf("%c", p->line[i]);
} else {
printf(" ");
for (j = 0; j < MAX_CONTEXT_LEN; j++) {
if (i+j < strlen(p->line) && !isspace(p->line[i+j])) {
printf("%c", p->line[i+j]);
} else {
break;
}
}
i += j-1;
}
}
printf("\n\n");
p = p->next;
}
}
int main() {
LineNode *lines = NULL;
char line[MAX_LINE_LEN];
while (fgets(line, MAX_LINE_LEN, stdin) != NULL) {
add_line(&lines, line);
}
print_kwic(lines);
return 0;
}
```
这个程序可以读入多行文本,然后对每一行进行KWIC索引,最后输出整个文本的KWIC索引结果。
### 回答2:
KWIC(Keyword-In-Context)索引系统是一种用于文本索引和检索的方法。在C语言中,可以通过链表来实现一个基本的KWIC索引系统。
首先,我们需要定义链表的节点结构。每个节点包含两个字段:第一个字段用于存储文本行,第二个字段用于指向下一个节点。可以使用以下代码定义节点结构:
```c
typedef struct Node {
char text[100];
struct Node* next;
} Node;
```
接下来,我们可以使用以下函数将文本行插入到链表中:
```c
void insert(Node** head, char text[]) {
Node* newNode = malloc(sizeof(Node));
strcpy(newNode->text, text);
newNode->next = *head;
*head = newNode;
}
```
然后,我们需要实现一个函数来读取多行文本并将其插入到链表中。可以使用如下代码:
```c
void readText(Node** head) {
char text[100];
printf("请输入文本行(输入exit退出):\n");
while (1) {
fgets(text, sizeof(text), stdin);
if (strcmp(text, "exit\n") == 0) {
break;
}
insert(head, text);
}
}
```
最后,我们可以实现一个函数来打印链表中的所有文本行,形成KWIC索引。可以使用如下代码:
```c
void printIndex(Node* head) {
printf("KWIC索引:\n");
while (head != NULL) {
printf("%s", head->text);
head = head->next;
}
}
```
此外,你还可以根据需求扩展这个基本的KWIC索引系统,例如实现对关键字的排序或添加检索功能等。
希望以上内容对你有所帮助。
阅读全文
相关推荐
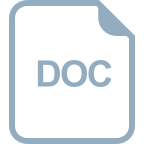
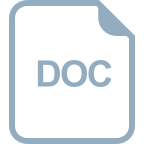
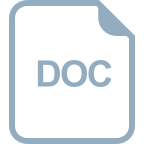
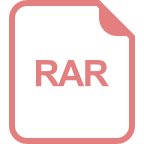
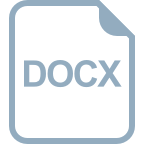
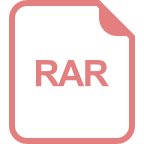
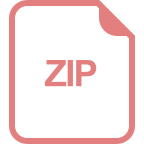
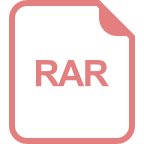
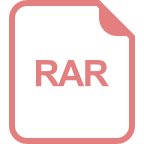
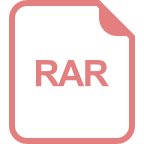
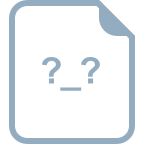
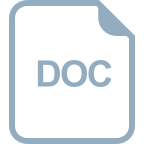
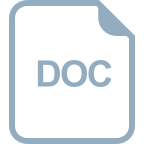
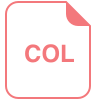
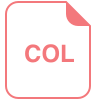
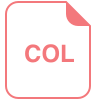
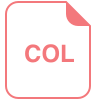