编写函数,判断用户输入的三个数字是否能构成三角形的三条边,要求在调用函数之前输入三个数字。
时间: 2024-11-25 17:16:28 浏览: 40
在编程中,你可以编写一个函数来检查给定的三个数字是否满足构成三角形的条件,即任意两边之和大于第三边。这种算法通常被称为“三角形不等式”。下面是一个简单的 Python 函数示例:
```python
def check_triangle(a, b, c):
# 检查每个数字是否大于0
if a <= 0 or b <= 0 or c <= 0:
return False, "所有边长必须大于0"
# 使用三角形不等式检查
if a + b > c and a + c > b and b + c > a:
return True, "可以构成三角形"
else:
return False, "不能构成三角形"
# 调用函数并获取结果
num1 = float(input("请输入第一个数:"))
num2 = float(input("请输入第二个数:"))
num3 = float(input("请输入第三个数:"))
result, message = check_triangle(num1, num2, num3)
print(f"能否构成三角形:{result}, {message}")
```
在这个例子中,用户需要依次输入三个数字,然后函数会检查这三个值是否能满足构成三角形的条件,并返回相应的信息。
相关问题
编写函数,判断用户输入的三个数字是否能构成三角形的三条边,要求在调用函数之前输入三个数字
当你需要编写一个函数来检查三个数是否能构成一个有效的三角形,你可以遵循以下步骤:
首先,我们需要理解三角形的性质:任意两边之和大于第三边。这意味着如果给定的三个数a、b和c满足这个条件,则它们可以组成一个三角形。
以下是一个简单的Python函数示例,它接受三个数作为参数并返回一个布尔值,表示它们能否形成一个三角形:
```python
def is_triangle(a, b, c):
# 检查任意两边之和是否大于第三边
if a + b > c and a + c > b and b + c > a:
return True
else:
return False
# 要使用此函数,你需要先获取用户输入
try:
num1 = float(input("请输入第一个数字:"))
num2 = float(input("请输入第二个数字:"))
num3 = float(input("请输入第三个数字:"))
except ValueError:
print("无效输入,请确保输入的是数字。")
else:
if is_triangle(num1, num2, num3):
print("这三个数可以构成三角形。")
else:
print("这三个数不能构成三角形。")
Python 编写函数判断用户输入的三个数字是否能构成三角形的三条边
好的,我可以帮你编写这个函数。以下是这个函数的 Python 代码:
```python
def is_triangle(a, b, c):
if a + b > c and a + c > b and b + c > a:
return True
else:
return False
```
这个函数接受三个参数,分别表示三角形的三条边的长度。它会判断这三个数字是否能构成一个三角形,如果能构成三角形,返回 True,否则返回 False。
你可以在调用这个函数时,传入你想要判断的三个数字,比如:
```python
print(is_triangle(3, 4, 5)) # True
print(is_triangle(1, 2, 3)) # False
```
希望这个函数对你有帮助!
阅读全文
相关推荐


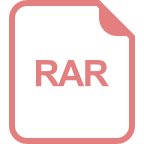














