java输入格式: 不需要用户输入,从主函数中为顺序表变量赋初值,其中总成绩由计算得到。 输出格式: 第一行输出表头,学号、姓名、数学成绩、语文成绩、物理成绩、总成绩。 之后将表变量初值按照学号、姓名、数学成绩、语文成绩、物理成绩、总成绩的顺序输出。
时间: 2024-09-14 13:09:46 浏览: 24
在Java中,要实现题目描述的输入和输出格式,可以创建一个类来表示学生的成绩信息,然后在主函数中创建这个类的对象,为对象的属性赋初值,计算总成绩,并按照指定格式输出。以下是一个简单的实现例子:
首先,定义一个学生类,包含学生的基本信息和成绩信息:
```java
public class Student {
private int id; // 学号
private String name; // 姓名
private int math; // 数学成绩
private int chinese; // 语文成绩
private int physics; // 物理成绩
private int total; // 总成绩
// 构造函数,用于初始化学生信息
public Student(int id, String name, int math, int chinese, int physics) {
this.id = id;
this.name = name;
this.math = math;
this.chinese = chinese;
this.physics = physics;
this.total = math + chinese + physics; // 计算总成绩
}
// 输出格式化信息的方法
public void printInfo() {
System.out.printf("%d\t%s\t%d\t%d\t%d\t%d\n", id, name, math, chinese, physics, total);
}
}
```
然后,在主函数中,创建`Student`对象的数组,为每个学生对象赋初值,并打印信息:
```java
public class Main {
public static void main(String[] args) {
// 创建学生对象数组
Student[] students = new Student[]{
new Student(1, "张三", 90, 85, 95),
new Student(2, "李四", 80, 88, 77),
// 可以添加更多的学生信息
};
// 打印表头
System.out.println("学号\t姓名\t数学成绩\t语文成绩\t物理成绩\t总成绩");
// 遍历数组,打印每个学生的信息
for (Student student : students) {
student.printInfo();
}
}
}
```
这段代码定义了一个`Student`类,并在其构造函数中计算总成绩。在`Main`类的`main`方法中创建了一个`Student`数组,并为每个学生对象初始化其属性值。之后遍历这个数组,调用每个学生对象的`printInfo`方法来按照指定的格式输出每个学生的信息。
相关推荐
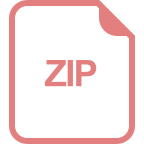
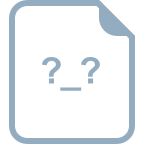
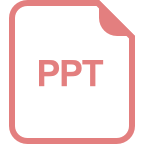














