java求数组最大值该值大于其左上、上、右上、左、右、左下、下、右下的值(如果有)
时间: 2024-10-07 20:07:15 浏览: 21
在Java中,如果你想要找到一个数组的最大值,同时满足它大于其周围八个相邻元素(如果存在),可以使用深度优先搜索(DFS)或者广度优先搜索(BFS)的方法,逐个比较当前元素和它的邻居。这里提供一个简单的DFS解决方案:
```java
import java.util.ArrayList;
import java.util.List;
class Solution {
int[][] directions = {{-1, -1}, {-1, 0}, {-1, 1},
{0, -1}, {0, 1},
{1, -1}, {1, 0}, {1, 1}};
public int findMaxWithNeighbors(int[][] array) {
if (array == null || array.length == 0) return Integer.MIN_VALUE;
int max = array[0][0];
dfs(array, 0, 0, max);
return max;
}
private void dfs(int[][] array, int row, int col, int currentMax) {
// Check neighbors and update max if necessary
for (int[] dir : directions) {
int newRow = row + dir[0], newCol = col + dir[1];
if (isValidIndex(newRow, newCol, array)) {
int neighborValue = array[newRow][newCol];
if (neighborValue > currentMax && neighborValue > array[row][col]) {
currentMax = neighborValue;
}
}
}
// Recursive call for the current cell's neighbors
for (int[] dir : directions) {
int newRow = row + dir[0], newCol = col + dir[1];
if (isValidIndex(newRow, newCol, array)) {
dfs(array, newRow, newCol, currentMax);
}
}
}
private boolean isValidIndex(int row, int col, int[][] array) {
return row >= 0 && row < array.length && col >= 0 && col < array[0].length;
}
}
```
这个`Solution`类里包含了`findMaxWithNeighbors`方法,它首先初始化最大值为第一个元素,然后通过递归深度优先地检查每个元素及其邻居是否符合条件。
阅读全文
相关推荐
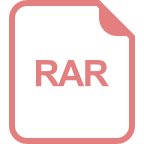
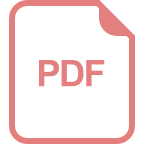
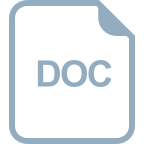
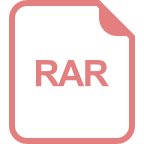
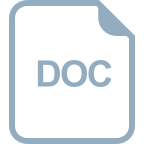
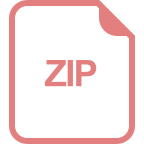
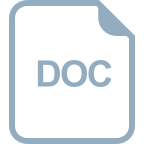
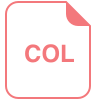
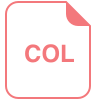







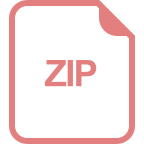