决策树c4.5算法python实现
时间: 2024-12-26 19:25:45 浏览: 5
### C4.5决策树算法简介
C4.5 是一种经典的决策树学习方法,由 Ross Quinlan 开发。该算法基于 ID3 进行改进,在处理连续属性、缺失值等方面表现更好。作为一种监督学习技术,它能够用于分类任务。
### 使用Python实现C4.5决策树
尽管Scikit-Learn库提供了ID3和CART模型的支持,但并没有直接提供C4.5的具体实现。然而,可以利用`sklearn.tree.DecisionTreeClassifier`类来构建类似的决策树结构,并通过自定义参数调整使其更接近于C4.5的行为[^1]。
为了更好地模拟C4.5的功能特性,下面给出了一种可能的方式:
#### 安装必要的依赖包
```bash
pip install numpy pandas scikit-learn matplotlib seaborn
```
#### 导入所需模块并准备数据集
```python
import numpy as np
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import LabelEncoder
from sklearn.tree import DecisionTreeClassifier
import matplotlib.pyplot as plt
import seaborn as sns
# 加载鸢尾花数据集作为示例
data = load_iris()
X = pd.DataFrame(data.data, columns=data.feature_names)
y = data.target
# 将标签编码成整数形式(如果必要的话)
le = LabelEncoder()
y = le.fit_transform(y)
# 划分训练集与测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
#### 构建类似于C4.5的决策树模型
```python
def build_c45_like_tree():
clf = DecisionTreeClassifier(
criterion='entropy', # 使用信息增益率代替原始的信息增益
splitter='best',
max_depth=None,
min_samples_split=2,
min_samples_leaf=1,
min_weight_fraction_leaf=0.,
max_features=None,
random_state=None,
max_leaf_nodes=None,
class_weight=None,
ccp_alpha=0.0
)
return clf
clf = build_c45_like_tree()
# 训练模型
clf.fit(X_train, y_train)
print(f'Training accuracy: {clf.score(X_train, y_train):.3f}')
print(f'Testing accuracy: {clf.score(X_test, y_test):.3f}')
# 可视化决策树
plt.figure(figsize=(12,8))
tree_plot = sns.scatterplot(x=X.iloc[:, 0], y=X.iloc[:, 1], hue=y, palette="deep", s=70)
for i in range(len(clf.tree_.feature)):
if clf.tree_.feature[i] != -2:
feature_name = list(X.columns)[clf.tree_.feature[i]]
threshold = clf.tree_.threshold[i]
plt.axvline(threshold, color='black', linestyle='dashed')
plt.show()
```
此代码片段展示了如何创建一个尽可能模仿C4.5特性的决策树实例。需要注意的是,由于缺乏对分裂标准的确切控制以及对于剪枝机制的不同设计,这并不能完全代表真正的C4.5算法行为[^2]。
阅读全文
相关推荐
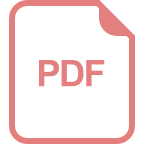
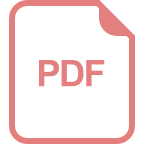
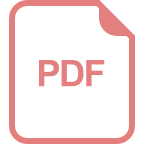






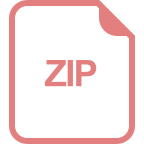






