python的魔术指令
时间: 2023-07-16 13:10:01 浏览: 64
Python的魔术指令是指在IPython交互式环境中可以使用的特殊命令。这些指令能够帮助用户更方便地控制交互式会话,执行一些特定的操作。以下是一些常用的Python魔术指令:
- `%run`: 运行外部Python脚本文件。
- `%load`: 加载外部Python脚本文件到交互式环境中。
- `%paste`: 将剪贴板中的代码粘贴到交互式环境中执行。
- `%reset`: 重置交互式环境,清除所有定义的变量和导入的模块。
- `%who`: 列出当前命名空间中定义的所有变量和模块。
- `%whos`: 更详细地列出当前命名空间中定义的所有变量和模块。
- `%time`: 测量单行代码的执行时间。
- `%timeit`: 多次运行一行代码,并计算平均执行时间。
- `%debug`: 进入交互式调试器,用于调试代码。
- `%history`: 显示交互式会话历史记录。
这些只是一些常见的魔术指令,IPython还提供了其他更多的魔术指令。你可以通过在IPython交互式环境中输入`%magic`来查看所有可用的魔术指令及其说明。
相关问题
python 初级指令
Python初级指令是指最基础的Python编程指令,用于实现简单的程序功能。下面是几个常用的Python初级指令:
1. 输出指令:使用print()函数可以将数据输出到屏幕上。例如,print("Hello World!")会在屏幕上输出"Hello World!"。这个指令对于调试和显示程序结果非常有用。
2. 输入指令:使用input()函数可以从用户那里接收输入。例如,name = input("请输入您的姓名:")会在屏幕上显示"请输入您的姓名:",然后等待用户输入并将输入值赋给变量name。
3. 变量赋值:使用赋值操作符"="可以将一个值赋给一个变量。例如,x = 5会将值5赋给变量x。
4. 条件语句:使用if语句可以根据条件选择性地执行代码块。例如,if x > 10:会判断变量x的值是否大于10,如果是,则执行if后的代码块。
5. 循环语句:使用for循环和while循环可以重复执行一段代码块。例如,for i in range(5):会执行循环体5次,每次将变量i设为0、1、2、3、4。而while循环会根据条件不断重复执行一段代码块,直到条件不再满足。
6. 数学运算:Python支持常见的数学运算,如加减乘除等。例如,x = 5 + 3会将8赋给变量x。
7. 字符串处理:Python提供了一系列处理字符串的方法,如拼接、切片、替换等。例如,s = "Hello" + "World!"会将"HelloWorld!"赋给变量s。
8. 函数定义:使用def关键字可以定义一个函数,函数是可重用的代码块。例如,def add(x, y):会定义一个函数add,接收两个参数x和y,并返回它们的和。
这些仅仅是Python初级指令的一小部分,但它们足够用来编写简单的程序。
python常用指令
Python常用指令包括:
1. 查看Python版本:您可以使用以下命令来查看您正在运行的Python版本:
- python -?
- python -h
- python --help
2. 查看Python版本号:您可以使用以下命令来查看Python的版本号:
- python -V
- python --version
3. 在终端上直接执行Python代码:如果您想在不打开和编辑.py文件的情况下直接从终端执行Python代码,可以使用以下命令标志:
- python -c "your_code"
4. 运行Python文件:如果您有一个.py文件,并且想要在终端运行它,可以使用以下命令:
- python filename.py
5. Python交互式模式:您可以在终端中直接进入Python交互式模式,以便逐行执行Python代码并查看结果。只需在终端中输入“python”即可进入交互式模式。在交互式模式下,您可以逐行输入和执行Python代码,并立即看到结果。
这些是Python的一些常用指令,它们可以帮助您在终端上执行Python代码和查看版本信息。同时,Python也是一门容易学习的编程语言,只需了解这些基本指令,就可以开始编写简单的Python程序。
相关推荐
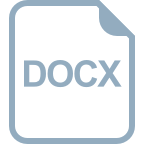












