springboot整合redis三主三从
时间: 2023-09-14 18:05:20 浏览: 191
在使用Spring Boot整合Redis时,可以实现主从复制的配置。主从复制是指在Redis集群中,有一个主节点负责处理写操作,并且将写操作同步到多个从节点上。这种配置可以提高系统的读取性能和可用性。
要实现Spring Boot整合Redis的主从复制,可以按照以下步骤进行操作:
1. 首先,在pom.xml文件中引入相关依赖。可以使用Spring Boot提供的`spring-boot-starter-data-redis`依赖来简化配置,同时需要引入`commons-pool2`依赖以支持连接池:
```
<!-- redis -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<!-- spring2.X集成redis所需common-pool2 -->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-pool2</artifactId>
<version>2.6.0</version>
</dependency>
```
2. 然后,在application.properties或application.yml文件中进行相关的配置。可以配置主节点和从节点的连接信息,例如:
```
# Redis主节点配置
spring.redis.host=主节点地址
spring.redis.port=主节点端口
spring.redis.password=主节点密码
# Redis从节点配置
spring.redis.sentinel.master=主节点名称
spring.redis.sentinel.nodes=从节点地址1,从节点地址2,从节点地址3
```
3. 最后,在Spring Boot的配置类中进行Redis的相关配置。可以使用`RedisSentinelConfiguration`类来配置主从节点的连接信息,例如:
```
@Configuration
public class RedisConfig {
@Value("${spring.redis.sentinel.master}")
private String master;
@Value("${spring.redis.sentinel.nodes}")
private String nodes;
@Bean
public RedisSentinelConfiguration redisSentinelConfiguration() {
RedisSentinelConfiguration configuration = new RedisSentinelConfiguration();
String[] nodeArray = nodes.split(",");
for (String node : nodeArray) {
String[] hostPort = node.split(":");
String host = hostPort<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [springboot整合redis代码](https://download.csdn.net/download/qq_34531925/10412347)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *2* [redis整合springboot](https://blog.csdn.net/weixin_45397764/article/details/118828384)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *3* [Java企业报表管理系统源码](https://download.csdn.net/download/m0_55416028/88269629)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 33.333333333333336%"]
[ .reference_list ]
阅读全文
相关推荐
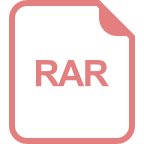
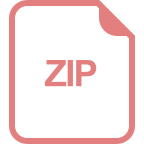
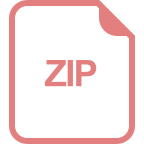
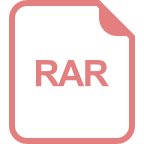
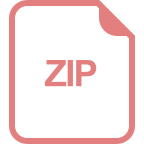

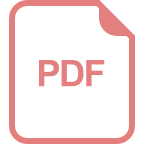
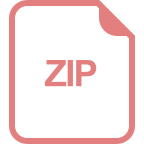
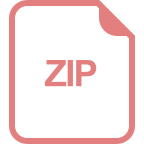
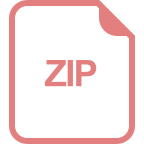
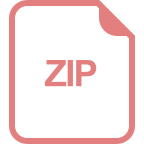