首先打印五个含有三个相同字符的字符串,要求字符是随机 的小写字符,打印不能换行,其次在第二行打印五个含有 3~5 个随机字符的字符串,其 中 3~5 是随机数据,字符也是随机字符,允许随机产生的字符不需要考虑重复问题
时间: 2024-10-16 12:03:11 浏览: 22
为了满足你的需求,我们可以使用Python的random库来生成随机小写字母。这里是一个简单的示例代码:
```python
import random
import string
# 生成五个含有三个相同字符的字符串
for _ in range(5):
char = random.choice(string.ascii_lowercase)
print(char * 3, end="")
print() # 换行,开始生成新的字符串
# 生成五个含有3到5个随机字符的字符串
for _ in range(5):
length = random.randint(3, 5)
chars = ''.join(random.sample(string.ascii_lowercase, length))
print(chars)
```
在这个代码中,`string.ascii_lowercase`包含了所有的小写字母。`random.choice`用于从字母集中选择一个字符,`* 3`表示复制该字符三次。`random.sample`则用于从字母集中无重复地选取指定长度的字符。
运行此代码,你会看到符合要求的字符串。
相关问题
编写程序,首先生成一个包含 1000 个随机字符的字符串(字符中含有英文 大、小写字母、数字和标点符号),返回一个元组,该元组第一个元素为标 点符号个数、第二个元素为数字字符个数、第三个元素为大写字母个数、第 四个元素为小写字母个数。
可以使用Python的random模块来生成随机字符串,然后使用字符串的内置方法来计算标点符号、数字字符、大写字母和小写字母的个数。下面是一个示例程序:
```python
import random
import string
def generate_random_string(length):
"""生成指定长度的随机字符串"""
# 可选字符集合,包含英文大、小写字母、数字和标点符号
chars = string.ascii_letters + string.digits + string.punctuation
# 从字符集合中随机选取指定数量的字符,拼接成字符串
return ''.join(random.choice(chars) for i in range(length))
def count_chars(string):
"""计算字符串中的标点符号、数字字符、大写字母和小写字母个数"""
punct_count = sum(1 for c in string if c in string.punctuation)
digit_count = sum(1 for c in string if c.isdigit())
upper_count = sum(1 for c in string if c.isupper())
lower_count = sum(1 for c in string if c.islower())
return (punct_count, digit_count, upper_count, lower_count)
# 生成随机字符串并计算字符个数
random_string = generate_random_string(1000)
char_counts = count_chars(random_string)
# 输出结果
print("标点符号个数:", char_counts[0])
print("数字字符个数:", char_counts[1])
print("大写字母个数:", char_counts[2])
print("小写字母个数:", char_counts[3])
```
这个程序中,首先定义了两个函数:`generate_random_string()`函数用于生成指定长度的随机字符串,`count_chars()`函数用于计算字符串中的标点符号、数字字符、大写字母和小写字母个数。然后在主程序中,先调用`generate_random_string()`函数生成一个长度为1000的随机字符串,并将其作为参数传递给`count_chars()`函数,最后输出计算结果。
输入一个以换行结束的字符串,统计并输出字符串中小写元音字母的个数
好的,这是一个计算型问题,我可以回答。请输入字符串:(输入完成后,我会统计小写元音字母的个数并输出)
请注意,输入的字符串中可以包含数字、符号、大写字母等其他字符,但我只会统计字符串中小写的元音字母个数。
阅读全文
相关推荐
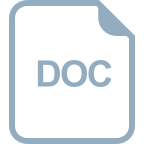
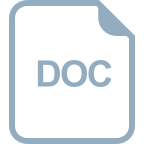
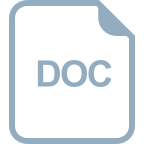


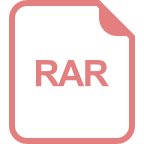
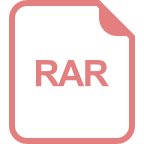
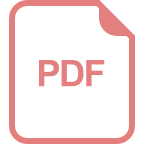







