在spyder中运行遗传算法
时间: 2024-02-10 08:05:17 浏览: 31
要在Spyder中运行遗传算法,您需要执行以下步骤:
1. 安装遗传算法库:您可以使用Python中的遗传算法库,例如DEAP或PyGMO。在Spyder中打开命令提示符并键入以下命令来安装DEAP:
```
pip install deap
```
或者键入以下命令来安装PyGMO:
```
pip install pygmo
```
2. 导入库:在Python脚本中导入所需的库,例如DEAP或PyGMO。
3. 定义适应度函数:编写适应度函数,该函数将评估种群中每个个体的适应度。适应度函数的输出必须是正数或零。如果适应度函数返回负值,则需要将其转换为正数。
4. 定义遗传算法参数:定义遗传算法的参数,例如种群大小,遗传算法的迭代次数,交叉概率,变异概率,选择算子等。
5. 定义遗传算法主循环:编写一个主循环来运行遗传算法。在每次迭代中,算法将选择最适应的个体,并对其进行交叉和变异操作,以生成新的个体。在每次迭代中,算法将计算适应度,并记录最佳个体的适应度和基因组。
6. 运行遗传算法:运行遗传算法,并查看结果。您可以使用Spyder的变量查看器来查看变量的值,例如最佳个体的适应度和基因组。
以下是一个简单的DEAP遗传算法示例:
```python
import random
from deap import base, creator, tools
# 定义适应度函数
def evaluate(individual):
return sum(individual),
# 定义遗传算法参数
pop_size = 50
gen_size = 100
cx_prob = 0.5
mut_prob = 0.2
# 创建遗传算法工具箱
toolbox = base.Toolbox()
# 创建一个布尔值个体(0或1)
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Individual", list, fitness=creator.FitnessMax)
toolbox.register("attr_bool", random.randint, 0, 1)
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_bool, n=10)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
# 注册遗传算法操作
toolbox.register("evaluate", evaluate)
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutFlipBit, indpb=0.05)
toolbox.register("select", tools.selTournament, tournsize=3)
# 运行遗传算法
pop = toolbox.population(n=pop_size)
for gen in range(gen_size):
# 评估种群
fitnesses = [toolbox.evaluate(ind) for ind in pop]
for ind, fit in zip(pop, fitnesses):
ind.fitness.values = fit
# 选择最适应的个体
offspring = toolbox.select(pop, len(pop))
# 复制选定的个体
offspring = list(map(toolbox.clone, offspring))
# 交叉和变异
for child1, child2 in zip(offspring[::2], offspring[1::2]):
if random.random() < cx_prob:
toolbox.mate(child1, child2)
del child1.fitness.values
del child2.fitness.values
for mutant in offspring:
if random.random() < mut_prob:
toolbox.mutate(mutant)
del mutant.fitness.values
# 评估变异后的个体
invalid_ind = [ind for ind in offspring if not ind.fitness.valid]
fitnesses = [toolbox.evaluate(ind) for ind in invalid_ind]
for ind, fit in zip(invalid_ind, fitnesses):
ind.fitness.values = fit
# 用变异后的个体替换种群
pop[:] = offspring
# 查看结果
best_ind = tools.selBest(pop, 1)[0]
print("Best individual:", best_ind)
print("Fitness:", best_ind.fitness.values[0])
```
相关推荐
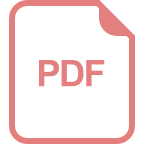
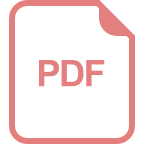
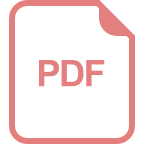














