SpringSecurity 自定义注解
时间: 2023-10-22 12:26:06 浏览: 123
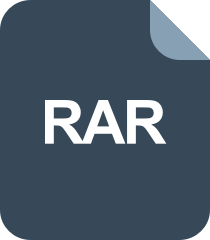
自定义注解
Spring Security允许我们通过自定义注解来标记方法或类,以便在授权过程中使用。下面是一个简单的示例:
1. 创建一个自定义注解:
```java
@Target({ElementType.METHOD, ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
public @interface CustomPermission {
String value() default "";
}
```
2. 在Spring Security配置类中,创建一个方法,用于解析自定义注解并应用相应的权限逻辑:
```java
@Configuration
@EnableGlobalMethodSecurity(prePostEnabled = true)
public class SecurityConfig extends WebSecurityConfigurerAdapter {
// ...其他配置...
@Override
protected MethodSecurityExpressionHandler createExpressionHandler() {
DefaultMethodSecurityExpressionHandler expressionHandler = new DefaultMethodSecurityExpressionHandler();
expressionHandler.setPermissionEvaluator(new CustomPermissionEvaluator());
return expressionHandler;
}
}
```
3. 创建一个自定义的 PermissionEvaluator 实现类,用于处理自定义注解中的权限逻辑:
```java
@Component
public class CustomPermissionEvaluator implements PermissionEvaluator {
@Override
public boolean hasPermission(Authentication authentication, Object targetDomainObject, Object permission) {
if (authentication == null || !authentication.isAuthenticated()) {
return false;
}
if (!(permission instanceof String)) {
return false;
}
// 在这里根据需要实现自定义的权限逻辑
return false;
}
@Override
public boolean hasPermission(Authentication authentication, Serializable targetId, String targetType, Object permission) {
// 该方法可用于处理非领域对象的权限逻辑,根据需要实现
return false;
}
}
```
4. 在需要授权的方法或类上使用自定义注解:
```java
@RestController
public class MyController {
@CustomPermission("customPermissionValue")
@GetMapping("/secured")
public String securedMethod() {
return "Hello, secured!";
}
}
```
通过以上步骤,我们可以使用自定义注解`@CustomPermission`来标记需要授权的方法或类,并在`CustomPermissionEvaluator`中实现具体的权限逻辑。然后,Spring Security会在授权过程中使用我们自定义的注解和权限逻辑。
阅读全文
相关推荐
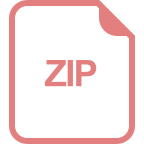
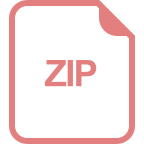
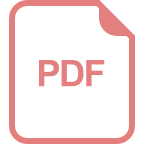
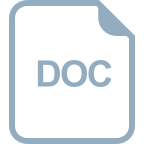



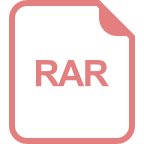
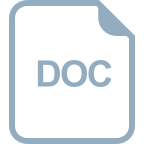
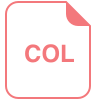





