nuxt 高德地图根据地址解析经纬度并展示位置
时间: 2023-12-14 17:38:00 浏览: 31
首先,你需要在高德地图开放平台申请一个 API Key,用于在你的应用程序中调用高德地图的 API。
然后,你可以使用高德地图的 JavaScript API 来实现根据地址解析经纬度并展示位置的功能。以下是一个简单的示例代码:
1. 在 nuxt.config.js 文件中添加高德地图的 API Key:
```javascript
module.exports = {
// ...
env: {
amapApiKey: 'your_amap_api_key'
}
// ...
}
```
2. 在你的组件中引入高德地图的 JavaScript API:
```javascript
import AMapLoader from '@amap/amap-jsapi-loader'
export default {
data() {
return {
map: null,
marker: null
}
},
async mounted() {
const AMap = await AMapLoader.load({
key: this.$config.amapApiKey,
version: '2.0',
plugins: ['AMap.Geocoder', 'AMap.Marker']
})
const address = '北京市朝阳区望京SOHO'
const geocoder = new AMap.Geocoder()
geocoder.getLocation(address, (status, result) => {
if (status === 'complete' && result.geocodes.length) {
const location = result.geocodes[0].location
this.map = new AMap.Map('map-container', {
center: location,
zoom: 16
})
this.marker = new AMap.Marker({
position: location,
map: this.map
})
} else {
console.error('Geocode failed:', status, result)
}
})
}
}
```
上述代码中,我们使用 AMapLoader 异步加载高德地图的 JavaScript API,并在组件的 mounted 钩子函数中调用 AMap.Geocoder 的 getLocation 方法来解析地址。如果解析成功,我们创建一个 AMap.Map 实例并在地图上添加一个 AMap.Marker 标记来展示位置。
3. 在模板中添加地图容器:
```html
<template>
<div>
<div id="map-container" style="width: 100%; height: 500px;"></div>
</div>
</template>
```
注意:上述代码中的 `address` 变量应该替换为你需要解析的地址。另外,如果你需要在组件销毁时清除地图,可以在 beforeDestroy 钩子函数中调用 `this.map.destroy()` 方法。
相关推荐
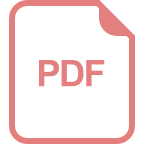
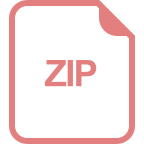














