如果使用线程池或进程池来控制,C语言怎么实现这个
时间: 2024-10-16 13:17:55 浏览: 23
在C语言中,特别是在POSIX环境中,你可以通过使用`pthreads`库来实现线程池。以下是一个简单的线程池概念的示例:
```c
#include <pthread.h>
#include <stdlib.h>
#define MAX_THREADS 60 // 线程池的最大数量
#define WORKERS_PER_THREAD 2 // 每个线程处理的任务数
typedef struct {
pthread_t thread_id;
int task_index;
} Worker;
// 线程池结构体
struct ThreadPool {
pthread_t threads[MAX_THREADS];
Worker workers[MAX_THREADS];
int num_threads;
bool running;
};
void* worker_thread(void* arg) {
Worker* worker = (Worker*)arg;
for (int i = 0; i < WORKERS_PER_THREAD && !thread_pool.running; ++i) {
// 这里处理客户端请求
client_request_handler(worker->task_index);
++worker->task_index;
}
return NULL;
}
ThreadPool* create_thread_pool() {
ThreadPool* pool = malloc(sizeof(ThreadPool));
if (!pool) {
perror("Memory allocation failed");
exit(EXIT_FAILURE);
}
pool->running = false;
for (int i = 0; i < MAX_THREADS; ++i) {
pool->workers[i].task_index = i * WORKERS_PER_THREAD;
pthread_create(&pool->threads[i], NULL, worker_thread, &pool->workers[i]);
}
pool->num_threads = MAX_THREADS;
return pool;
}
void start_thread_pool(ThreadPool* pool) {
pool->running = true;
for (int i = 0; i < pool->num_threads; ++i)
pthread_join(pool->threads[i], NULL);
}
void stop_thread_pool(ThreadPool* pool) {
pool->running = false;
// 可能还需要加入同步机制来确保所有线程完成当前任务
}
```
在这个例子中,`create_thread_pool`函数初始化线程池,`start_thread_pool`启动线程,`stop_thread_pool`关闭线程池。每个线程会处理WORKERS_PER_THREAD个请求,直到线程池停止。
注意,C语言本身并不内置线程池功能,上述代码仅提供了一个基础框架,实际应用中可能需要更复杂的数据同步和错误处理机制。
阅读全文
相关推荐
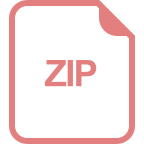
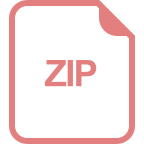
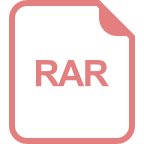

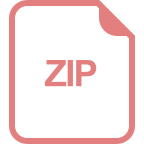
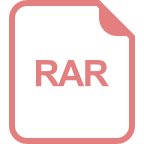
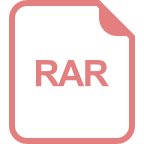
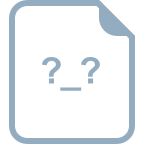
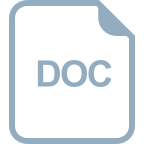
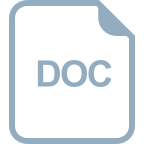
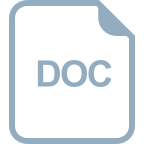
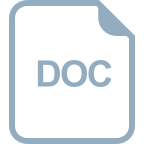
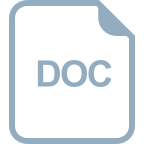
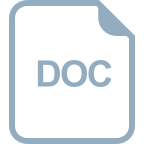
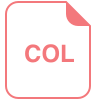
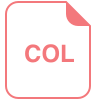
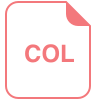
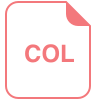
