DS18B20.H和DS18B20.C是什么
时间: 2024-04-28 08:24:07 浏览: 138
DS18B20.H和DS18B20.C是DS18B20数字温度传感器的头文件和源文件,它们是用于C语言编写的程序文件。DS18B20是一种数字式温度传感器,可通过1线接口实现与微控制器的通信。DS18B20.H和DS18B20.C文件提供了一些函数和定义,可以方便地对DS18B20进行读取和写入操作,用于在嵌入式系统中实现温度检测和控制等功能。
相关问题
DS18B20.C和ds18b20.h怎么写
DS18B20是一种数字温度传感器,需要通过单总线协议进行通讯。下面是一个简单的DS18B20代码示例,包含DS18B20.C和DS18B20.H两个文件。
DS18B20.H文件:
```c
#ifndef __DS18B20_H__
#define __DS18B20_H__
#include "stm32f4xx_hal.h"
#define DS18B20_PORT GPIOA
#define DS18B20_PIN GPIO_PIN_0
void DS18B20_Init(void);
void DS18B20_Start(void);
void DS18B20_Write(uint8_t data);
uint8_t DS18B20_Read(void);
float DS18B20_GetTemperature(void);
#endif
```
DS18B20.C文件:
```c
#include "ds18b20.h"
void DS18B20_Init(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
__HAL_RCC_GPIOA_CLK_ENABLE();
GPIO_InitStruct.Pin = DS18B20_PIN;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_OD;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH;
HAL_GPIO_Init(DS18B20_PORT, &GPIO_InitStruct);
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_SET);
}
void DS18B20_Start(void)
{
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_RESET);
HAL_Delay(480);
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_SET);
HAL_Delay(60);
}
void DS18B20_Write(uint8_t data)
{
for (int i = 0; i < 8; i++)
{
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_RESET);
HAL_Delay(1);
if ((data >> i) & 0x01)
{
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_SET);
}
HAL_Delay(60);
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_SET);
}
}
uint8_t DS18B20_Read(void)
{
uint8_t data = 0x00;
for (int i = 0; i < 8; i++)
{
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_RESET);
HAL_Delay(1);
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_SET);
HAL_Delay(15);
if (HAL_GPIO_ReadPin(DS18B20_PORT, DS18B20_PIN))
{
data |= 0x01 << i;
}
HAL_Delay(45);
}
return data;
}
float DS18B20_GetTemperature(void)
{
uint8_t temp_l, temp_h;
float temperature;
DS18B20_Start();
DS18B20_Write(0xCC);
DS18B20_Write(0x44);
HAL_Delay(750);
DS18B20_Start();
DS18B20_Write(0xCC);
DS18B20_Write(0xBE);
temp_l = DS18B20_Read();
temp_h = DS18B20_Read();
temperature = (temp_h << 8) + temp_l;
temperature = temperature * 0.0625;
return temperature;
}
```
这段代码使用了STM32 HAL库来实现GPIO的控制,需要根据具体的硬件平台进行修改。函数DS18B20_GetTemperature()用于读取温度值,其他函数用于实现单总线协议的通信。
stm32f10x.h编写ds18b20.c和ds18b20.h
以下是一个简单的DS18B20读取温度值的代码示例,其中使用了STM32F10x标准库函数。需要注意的是,这里使用了单总线模式,即将DS18B20的数据线与STM32的GPIO口相连,没有使用额外的硬件电路。
ds18b20.h文件:
```c
#ifndef __DS18B20_H
#define __DS18B20_H
#include "stm32f10x.h"
#define DS18B20_GPIO_PORT GPIOA
#define DS18B20_GPIO_PIN GPIO_Pin_0
float DS18B20_GetTemperature(void);
#endif /* __DS18B20_H */
```
ds18b20.c文件:
```c
#include "ds18b20.h"
/* DS18B20复位 */
static void DS18B20_Reset(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = DS18B20_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DS18B20_GPIO_PORT, &GPIO_InitStructure);
GPIO_ResetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
delay_us(500);
GPIO_SetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
delay_us(80);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(DS18B20_GPIO_PORT, &GPIO_InitStructure);
}
/* 发送字节数据 */
static void DS18B20_WriteByte(uint8_t data)
{
uint8_t i = 0;
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = DS18B20_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DS18B20_GPIO_PORT, &GPIO_InitStructure);
for (i = 0; i < 8; i++)
{
if ((data >> i) & 0x01)
{
GPIO_ResetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
delay_us(1);
GPIO_SetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
delay_us(80);
}
else
{
GPIO_ResetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
delay_us(80);
GPIO_SetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
delay_us(1);
}
}
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(DS18B20_GPIO_PORT, &GPIO_InitStructure);
}
/* 读取字节数据 */
static uint8_t DS18B20_ReadByte(void)
{
uint8_t i = 0, data = 0;
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = DS18B20_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DS18B20_GPIO_PORT, &GPIO_InitStructure);
for (i = 0; i < 8; i++)
{
GPIO_ResetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
delay_us(4);
GPIO_SetBits(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN);
delay_us(10);
if (GPIO_ReadInputDataBit(DS18B20_GPIO_PORT, DS18B20_GPIO_PIN))
{
data |= 0x01 << i;
}
delay_us(50);
}
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(DS18B20_GPIO_PORT, &GPIO_InitStructure);
return data;
}
/* 获取温度值 */
float DS18B20_GetTemperature(void)
{
uint8_t temp_l = 0, temp_h = 0;
float temperature = 0.0;
DS18B20_Reset();
DS18B20_WriteByte(0xCC);
DS18B20_WriteByte(0x44);
delay_ms(800);
DS18B20_Reset();
DS18B20_WriteByte(0xCC);
DS18B20_WriteByte(0xBE);
temp_l = DS18B20_ReadByte();
temp_h = DS18B20_ReadByte();
temperature = (float)((int16_t)(temp_h << 8 | temp_l)) / 16.0;
return temperature;
}
```
其中,delay_us和delay_ms函数为延时函数,需要自行实现。可以参考下面的示例:
```c
void delay_us(uint32_t time)
{
uint32_t i = 0;
for (i = 0; i < time * 8; i++);
}
void delay_ms(uint32_t time)
{
uint32_t i = 0;
for (i = 0; i < time * 8000; i++);
}
```
此外,需要在主函数中调用库函数GPIO_Init和RCC_APB2PeriphClockCmd等函数初始化STM32的GPIO口和时钟。具体使用方法可以参考STM32F10x官方文档和示例代码。
阅读全文
相关推荐
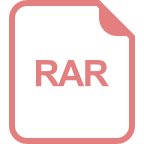
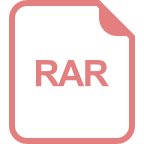













