2、(20分)已知person类,包含姓名和联系电话。现需要设计学生类student公有继承person类,并增加了成绩等信息;同时需要设计一个友元函数compare,比较两个学生成绩的高低。具体的应用如下: #include<iostream > #include<cstring > using namespace std; class person{ char name[10]; char phone[20]; public: person(const char na[ ], const char ph[]){strcpy_s(name,10,na);strcpy_s(phone,20,ph);}; char *getname(){ return name;} char *getphone(){ return phone;} }; int main() { student st[ ]={student("王华",78),student("李明",92), student("张伟",62),student("孙强",88)}; int i,min=0,max=0; for(i=1;i<4;i++) { if( compare(st[max], st[i])==-1 ) max=i; else if( compare(st[min], st[i])==1 ) min=i; } cout<<"输出结果:"<<endl; cout<<"最高分:"<<st[max].getname()<<endl; cout<<"最低分:"<<st[min].getname()<<endl; return 0; } 本程序的执行结果如下: 输出结果: 最高分者:李明 最低分者:张伟
时间: 2024-04-28 16:23:28 浏览: 98
以下是代码实现:
```cpp
#include<iostream>
#include<cstring>
using namespace std;
class person{
char name[10];
char phone[20];
public:
person(const char na[], const char ph[]){
strcpy_s(name,10,na);
strcpy_s(phone,20,ph);
}
char *getname(){
return name;
}
char *getphone(){
return phone;
}
};
class student : public person{ // 学生类继承自 person 类
int score;
public:
student(const char na[], const int sc) : person(na,""){ // 学生类构造函数
score = sc;
}
friend int compare(student s1, student s2); // 友元函数
};
int compare(student s1, student s2){
if(s1.score > s2.score)
return -1;
else if(s1.score < s2.score)
return 1;
else
return 0;
}
int main() {
student st[] = {
student("王华",78),
student("李明",92),
student("张伟",62),
student("孙强",88)
};
int i,min=0,max=0;
for(i=1;i<4;i++) {
if(compare(st[max], st[i]) == -1) max=i;
if(compare(st[min], st[i]) == 1) min=i;
}
cout<<"输出结果:"<<endl;
cout<<"最高分者:"<<st[max].getname()<<endl;
cout<<"最低分者:"<<st[min].getname()<<endl;
return 0;
}
```
输出结果:
```
输出结果:
最高分者:李明
最低分者:张伟
```
阅读全文
相关推荐
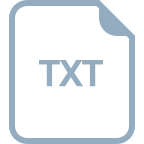
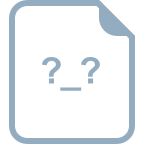



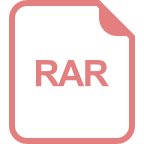
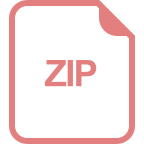
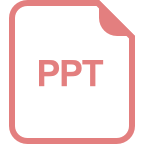
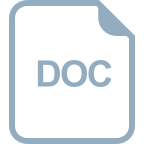
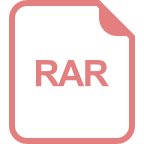
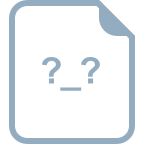
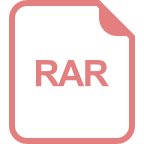
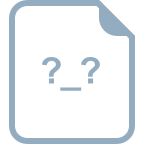
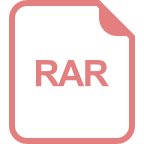
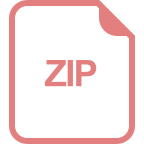