rsa加密算法c++语言代码
时间: 2023-11-08 21:10:52 浏览: 48
以下是RSA加密算法的C++语言代码示例:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
int gcd(int a, int b) {
if (b == 0) {
return a;
}
return gcd(b, a % b);
}
int modPow(int base, int exponent, int modulus) {
int result = 1;
while (exponent > 0) {
if (exponent % 2 == 1) {
result = (result * base) % modulus;
}
base = (base * base) % modulus;
exponent = exponent / 2;
}
return result;
}
int main() {
int p, q, n, phi, e, d, message, ciphertext, plaintext;
// Step 1: Choose two prime numbers
p = 17;
q = 19;
// Step 2: Compute n = p * q
n = p * q;
// Step 3: Compute phi(n) = (p - 1) * (q - 1)
phi = (p - 1) * (q - 1);
// Step 4: Choose an integer e such that 1 < e < phi(n)
e = 5;
while (gcd(e, phi) != 1) {
e++;
}
// Step 5: Compute d such that d * e = 1 (mod phi(n))
d = 1;
while ((d * e) % phi != 1) {
d++;
}
// Step 6: Encrypt the message using the public key (e, n)
message = 123;
ciphertext = modPow(message, e, n);
cout << "Encrypted message: " << ciphertext << endl;
// Step 7: Decrypt the ciphertext using the private key (d, n)
plaintext = modPow(ciphertext, d, n);
cout << "Decrypted message: " << plaintext << endl;
return 0;
}
```
该示例实现了RSA加密算法的核心步骤,包括:
1. 选择两个质数 p 和 q
2. 计算 n = p * q
3. 计算 phi(n) = (p - 1) * (q - 1)
4. 选择一个整数 e 使得 1 < e < phi(n),且 gcd(e, phi(n)) = 1
5. 计算 d 使得 d * e = 1 (mod phi(n))
6. 使用公钥 (e, n) 加密消息
7. 使用私钥 (d, n) 解密密文
在实际应用中,需要考虑到安全性和性能等因素,例如选择更大的质数和密钥长度等。
相关推荐
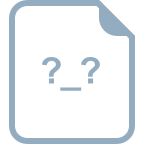
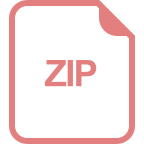














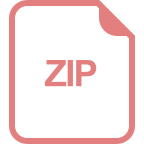