用jQuery写一个轮播图带控件的代码并解释
时间: 2024-05-15 20:15:07 浏览: 72
以下是一个简单的使用jQuery实现轮播图的例子:
HTML代码:
```html
<div class="slider">
<ul class="slides">
<li><img src="image1.jpg"></li>
<li><img src="image2.jpg"></li>
<li><img src="image3.jpg"></li>
</ul>
<div class="controls">
<span class="prev">‹</span>
<span class="next">›</span>
</div>
</div>
```
CSS代码:
```css
.slider {
position: relative;
overflow: hidden;
}
.slides {
list-style: none;
margin: 0;
padding: 0;
position: relative;
}
.slides li {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
opacity: 0;
z-index: 1;
transition: opacity 1s ease-in-out;
}
.slides li:first-child {
position: relative;
opacity: 1;
z-index: 2;
}
.controls {
position: absolute;
bottom: 10px;
left: 50%;
transform: translateX(-50%);
}
.controls span {
display: inline-block;
width: 30px;
height: 30px;
line-height: 30px;
text-align: center;
background-color: rgba(255, 255, 255, 0.8);
border-radius: 50%;
cursor: pointer;
font-size: 20px;
color: #333;
margin: 0 10px;
transition: background-color 0.5s ease-in-out;
}
.controls span:hover {
background-color: rgba(255, 255, 255, 1);
}
```
JavaScript代码:
```javascript
$(document).ready(function() {
var $slider = $('.slider');
var $slides = $slider.find('.slides li');
var $controls = $slider.find('.controls span');
var currentIndex = 0;
function goToSlide(index) {
$slides.eq(currentIndex).css('z-index', 1);
$slides.eq(index).css('z-index', 2);
$slides.eq(currentIndex).animate({ opacity: 0 }, 1000);
$slides.eq(index).animate({ opacity: 1 }, 1000);
currentIndex = index;
}
$controls.on('click', function() {
if ($(this).hasClass('prev')) {
var index = (currentIndex == 0) ? $slides.length - 1 : currentIndex - 1;
goToSlide(index);
} else {
var index = (currentIndex == $slides.length - 1) ? 0 : currentIndex + 1;
goToSlide(index);
}
});
});
```
这个例子中的轮播图实现了以下功能:
1. 轮播图可以自动播放,每隔一段时间切换到下一张图片。
2. 轮播图可以手动切换到上一张或下一张图片。
3. 轮播图下方有控件,可以点击控件切换到对应的图片。
这个轮播图的原理是利用CSS的`position: absolute`属性和jQuery的`animate()`方法来实现图片的切换效果。每个图片都是绝对定位的,重叠在一起,只有当前图片的`z-index`值为2,其他图片的`z-index`值为1。切换图片时,先将当前图片的`z-index`值设为1,将目标图片的`z-index`值设为2,然后通过`animate()`方法将当前图片的透明度从1渐变到0,将目标图片的透明度从0渐变到1。切换图片时还需要更新当前图片的索引值。控件的实现是通过jQuery的`on()`方法给控件绑定`click`事件来实现的。
阅读全文
相关推荐
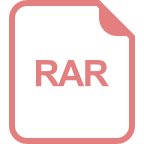
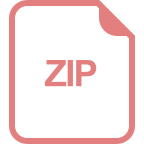
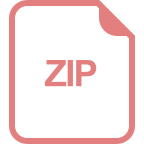









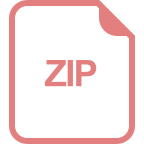
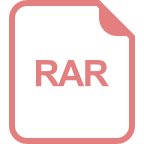
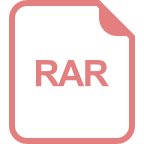
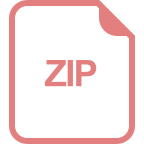
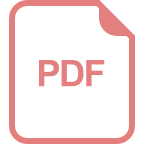
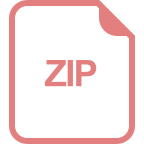
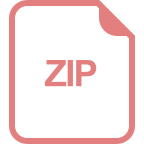